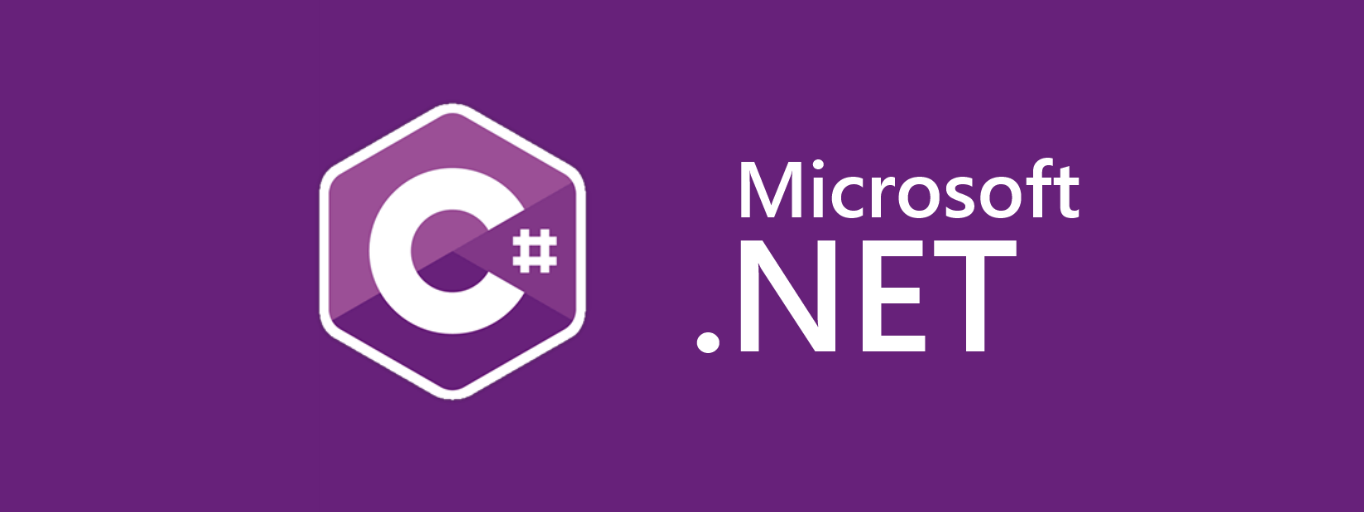
현재는 아래와 같이 고정된 데이터를 넘기지만 가변의 데이터를 어떻게 처리하는지 알아보자
// ...
class PlayerInfoReq : Packet
{
public long playerId;
// ...
우선 약간의 코드정리를 해보자
public override ArraySegment<byte> Write()
{
ArraySegment<byte> segment = SendBufferHelper.Open(4096);
ushort size = 0;
bool success = true;
// Span을 이용
Span<byte> s = new Span<byte>(segment.Array, segment.Offset, segment.Count);
size += sizeof(ushort);
success &= BitConverter.TryWriteBytes(s.Slice(size, s.Length - size), this.packetId);
size += sizeof(ushort);
success &= BitConverter.TryWriteBytes(s.Slice(size, s.Length - size), this.playerId);
size += sizeof(long);
success &= BitConverter.TryWriteBytes(s, size);
if (success == false)
return null;
return SendBufferHelper.Close(size);
}
public override void Read(ArraySegment<byte> segment)
{
ushort count = 0;
ReadOnlySpan<byte> s = new ReadOnlySpan<byte>(segment.Array, segment.Offset, segment.Count);
count += 2; // size
count += 2; // id
this.playerId = BitConverter.ToInt64(s.Slice(count, s.Length - count));
count += 8;
}
// ...
public override ArraySegment<byte> Write()
{
ArraySegment<byte> segment = SendBufferHelper.Open(4096);
ushort size = 0;
bool success = true;
Span<byte> s = new Span<byte>(segment.Array, segment.Offset, segment.Count);
size += sizeof(ushort);
success &= BitConverter.TryWriteBytes(s.Slice(size, s.Length - size), this.packetId);
size += sizeof(ushort);
success &= BitConverter.TryWriteBytes(s.Slice(size, s.Length - size), this.playerId);
size += sizeof(long);
// string
// string의 len을 먼저보내고 내용을 byte로 보내자
ushort nameLen = (ushort)Encoding.Unicode.GetByteCount(this.name);
success &= BitConverter.TryWriteBytes(s.Slice(size, s.Length - size), nameLen);
size += sizeof(ushort);
Array.Copy(Encoding.Unicode.GetBytes(this.name), 0, segment.Array, size, nameLen);
size += nameLen;
success &= BitConverter.TryWriteBytes(s, size);
// ...
public override void Read(ArraySegment<byte> segment)
{
ushort count = 0;
ReadOnlySpan<byte> s = new ReadOnlySpan<byte>(segment.Array, segment.Offset, segment.Count);
count += sizeof(ushort); // size
count += sizeof(ushort); // id
this.playerId = BitConverter.ToInt64(s.Slice(count, s.Length - count));
count += sizeof(long);
// string
ushort nameLen = (ushort)BitConverter.ToInt64(s.Slice(count, s.Length - count));
count += sizeof(ushort);
this.name = Encoding.Unicode.GetString(s.Slice(count, nameLen));
}