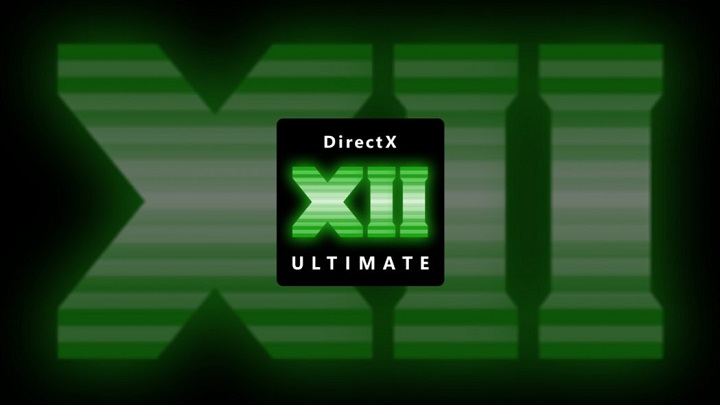
우선 간단히 렌더링 파이프라인에 대해 설명
- Input-Assembler Stage : 정점정보(삼각형이 어떠한 좌표에 있는지)를 관리
- Vertex Shader Stage : 정점정보를 연산(좌표이동 등)
- Tessellation Stage : 새로운 정점을 계산(경우에 따라 정점이 추가/제거되어야 할 필요가 있음(Ex> 멀리있는 산의 표현))
- Geometry Shader Stage : 새로운 정점을 계산
- Rasterize Stage : 여기부터 색상을 입힌다, 정점의 색상 정보 관리(A, B점으로 가며 색상을 어떻게 변경하는지 정점간 선을 어떻게 그을지 등)
- Pixel Shader Stage : 색상을 넣는다
- Output-Merger Stage : 모든 색상을 조합
일단 이런게 있다고만 알고 있자.
VS2019로 실행할 것.
프로젝트 만들기
Windows 데스크톱 애플리케이션
메시지 루프 구조를 변경한다.
// 기본 메시지 루프입니다:
while (true)
{
// 메시지가 들어올때만 받는게 아니라 있는지를 체크후 꺼내는 형태로 변경
if (PeekMessage(&msg, nullptr, 0, 0, PM_REMOVE))
{
if (msg.message == WM_QUIT)
break;
if (!TranslateAccelerator(msg.hwnd, hAccelTable, &msg))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
}
// TODO : 게임로직
}
미리 컴파일된 헤더를 사용하게 변경
// pch.h
#pragma once
#include <vector>
#include <memory>
using namespace std;
Game class 만들기
#pragma once
class Game
{
public:
void Init();
void Update();
};
#include "pch.h"
#include "Game.h"
void Game::Init()
{
}
void Game::Update()
{
}
Game을 주기적 호출
MSG msg;
//Game* game = new Game();
unique_ptr<Game> game = make_unique<Game>();
game->Init();
// 기본 메시지 루프입니다:
while (true)
{
if (PeekMessage(&msg, nullptr, 0, 0, PM_REMOVE))
{
if (msg.message == WM_QUIT)
break;
if (!TranslateAccelerator(msg.hwnd, hAccelTable, &msg))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
}
// TODO : 게임로직
game->Update();
}
Direct 라이브러리를 관리하는 프로젝트를 하나 더 생성
정적라이브러리로 생성
d3dx12.h
(MS에서 제공하는 라이브러리기능 모음)를 추가- 미리 컴파일된 헤더에 아래를 추가
#pragma once
// 각종 include
#include <windows.h>
#include <tchar.h>
#include <memory>
#include <string>
#include <vector>
#include <array>
#include <list>
#include <map>
using namespace std;
#include "d3dx12.h"
#include <d3d12.h>
#include <wrl.h>
#include <d3dcompiler.h>
#include <dxgi.h>
#include <DirectXMath.h>
#include <DirectXPackedVector.h>
#include <DirectXColors.h>
using namespace DirectX;
using namespace DirectX::PackedVector;
using namespace Microsoft::WRL;
// 각종 lib
#pragma comment(lib, "d3d12")
#pragma comment(lib, "dxgi")
#pragma comment(lib, "dxguid")
#pragma comment(lib, "d3dcompiler")
// 각종 typedef
using int8 = __int8;
using int16 = __int16;
using int32 = __int32;
using int64 = __int64;
using uint8 = unsigned __int8;
using uint16 = unsigned __int16;
using uint32 = unsigned __int32;
using uint64 = unsigned __int64;
using Vec2 = XMFLOAT2;
using Vec3 = XMFLOAT3;
using Vec4 = XMFLOAT4;
using Matrix = XMMATRIX;
void HelloEngine();