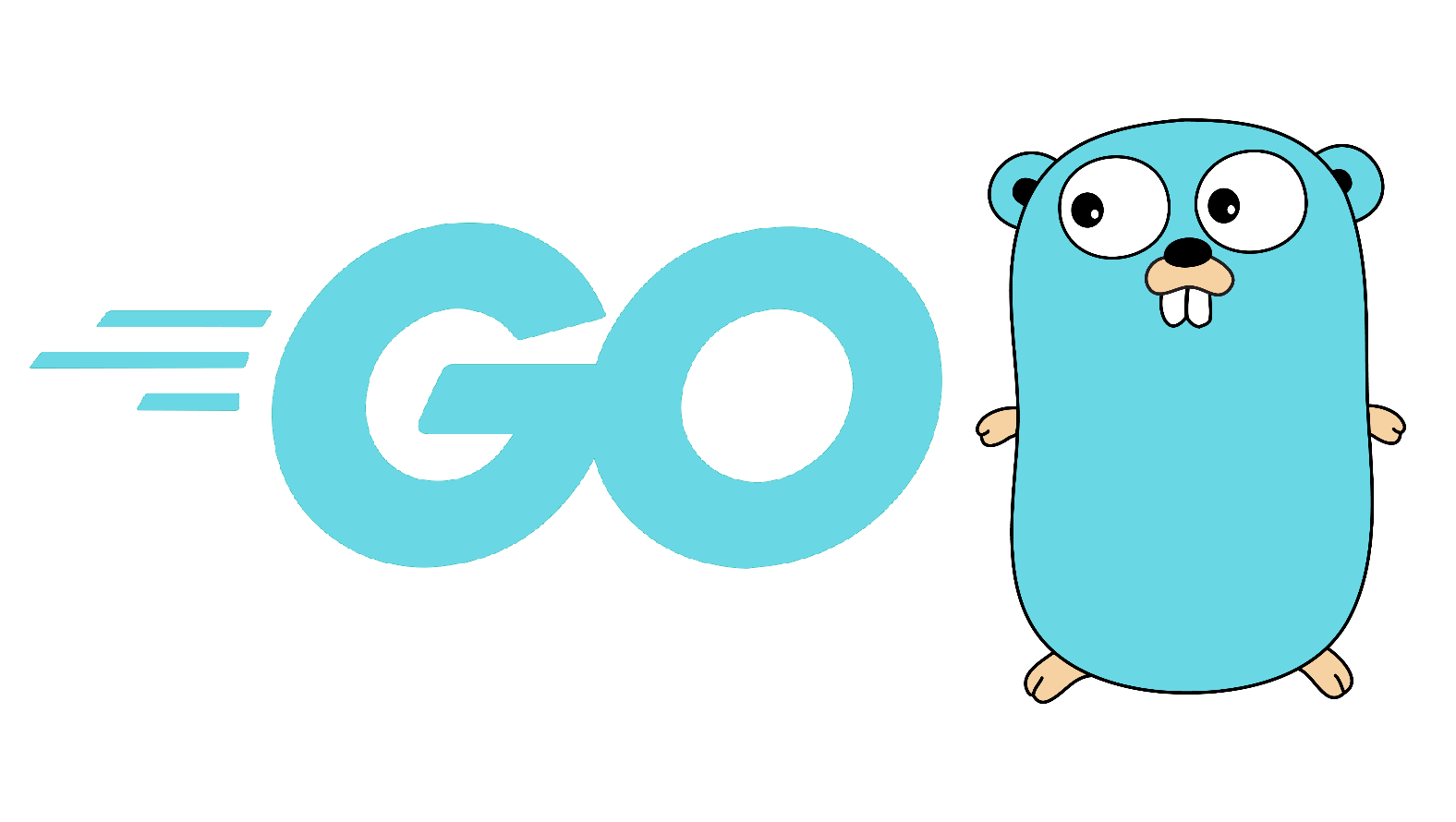
package main
import "fmt"
// 사용자 정의 타입(구조체)
type Car struct {
name string
color string
price int64
tax int64
}
//구조체 <-> 메소드 바인딩
func (c Car) Price() int64 {
return c.price + c.tax
}
func main() {
//Go -> 객체 지향 타입을 구조체로 정의한다.(클래스, 상속 개념 없음)
//상태, 메소드를 분리해서 정의(결합성 없음)
//사용자 정의 타입 : 구조체 , 인터페이스 , 기본타입 , 함수
//구조체와 -> 메서드 연결을 통해서 타 언어의 클래스 형식 처럼 사용 가능(객체 지향)
//예제1
bmw := Car{name: "520d", price: 54500000, color: "white", tax: 545000}
benz := Car{name: "220d", price: 74500000, color: "white", tax: 745000}
bmwPrice := bmw.Price()
benzPrice := benz.Price()
fmt.Println("ex1 : ", &bmw)
fmt.Println("ex1 : ", bmwPrice)
fmt.Println("ex1 : ", bmw.Price())
fmt.Println("ex1 : ", &benz == &bmw)
fmt.Println("ex1 : ", &benz)
fmt.Println("ex1 : ", benzPrice)
fmt.Println("ex1 : ", benz.Price())
}
package main
import "fmt"
type cnt int
func main() {
//기본 자료형 사용자 정의 타입
//예제1
a := cnt(5)
fmt.Println("ex1 : ", a)
//예제2
var b cnt = 15
fmt.Println("ex2 : ", b)
//testConvertT(b) //예외 발생 (중요!) 사용자 정의 타입 <-> 기본 타입 : 매개변수 전달 시에 변환해야 사용 가능 (cnt(5), int(5))
testConvertT(int(b))
testConvertD(b)
testConvertD(cnt(10)) //사용 가능
//testConvertD(int(b)) //예외 발생 (중요!) 사용자 정의 타입 <-> 기본 타입 : 매개변수 전달 시에 변환해야 사용 가능 (cnt(5), int(5))
}
func testConvertT(i int) {
fmt.Println("ex 2 : (Default Type) : ", i)
}
func testConvertD(i cnt) {
fmt.Println("ex 2 : (Custom Type) : ", i)
}
package main
import "fmt"
type totCost func(int, int) int
func describe(cnt int, price int, fn totCost) {
fmt.Printf("cnt: %d, price: %d, orderPrice: %d", cnt, price, fn(cnt, price))
}
func main() {
//함수 사용자 정의 타입
//예제1
var orderPrice totCost
orderPrice = func(cnt int, price int) int {
return (cnt * price) + 1000000
}
describe(3, 10000000, orderPrice)
}
package main
import "fmt"
type shopingBasket struct{ cnt, price int }
func (b shopingBasket) purchase() int {
return b.cnt * b.price
}
func (b *shopingBasket) rePurchaseP(cnt, price int) {
b.cnt += cnt
b.price += price
}
func (b shopingBasket) rePurchaseD(cnt, price int) {
b.cnt += cnt
b.price += price
}
func main() {
//리시버 전달(값, 참조) 형식
//함수는 기본적으로 값 호출 -> 변수의 값이 복사 후 내부 전달(원본 수정X) -> 맵, 슬라이스 등은 참조 전달
//리시버(구조체)도 마찬가지로 포인터를 활용해서 메소드 내에서 원본 수정 가능
//예제1
bs1 := shopingBasket{3, 5000}
fmt.Println("ex1(totPrice) : ", bs1.purchase())
bs1.rePurchaseP(10, 10000) //매개변수 전달(참조)
fmt.Println("ex1(totPrice) :", bs1.purchase())
fmt.Println()
//예제2
bs2 := shopingBasket{5, 5000}
fmt.Println("ex2(totPrice) : ", bs2.purchase())
bs2.rePurchaseD(10, 10000) //매개변수 전달(복사)
fmt.Println("ex2(totPrice) :", bs2.purchase())
fmt.Println()
//예제3
bs3 := shopingBasket{10, 10000}
fmt.Println("ex3(totPrice) : ", bs3.purchase())
bs3.rePurchaseP(-5, -7000) //매개변수 전달(참조)
fmt.Println("ex3(totPrice) :", bs3.purchase())
}