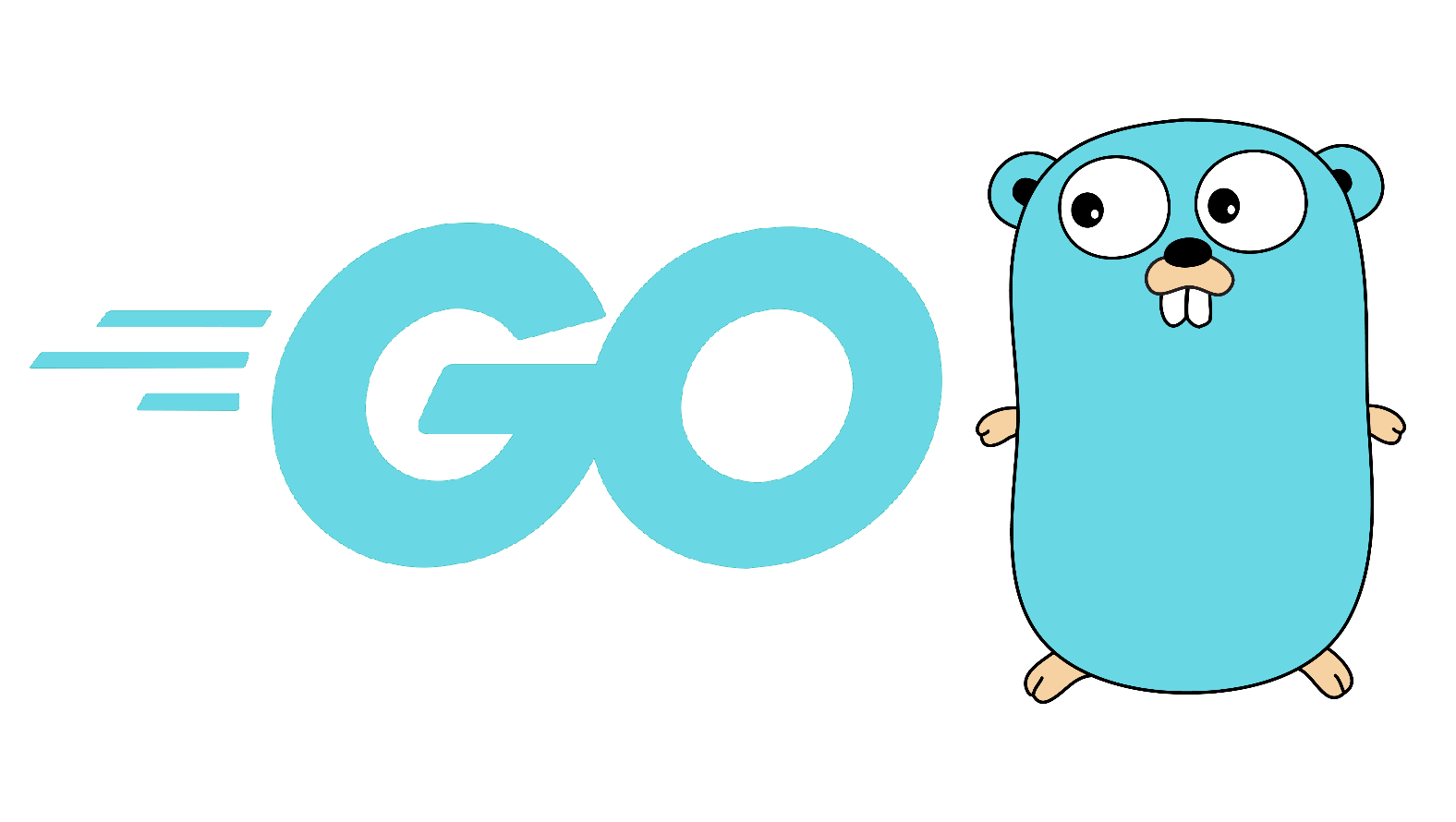
package main
import "fmt"
func main() {
//맵(Map)
//맵 : 해시테이블, 딕셔너리(파이썬), Key-Value로 자료 저장
//레퍼런스 타입!(참조 값 전달)
//비교 연산자 사용 불가능(참조 타입이므로)
//특징 : 참조타입 키(Key)로 사용 불가능 , 값(Value)으로 모든 타입 사용 가능
//make 함수 및 축약(리터럴)로 초기화 가능
//순서 없음
//예제1
var map1 map[string]int = make(map[string]int) //정석
var map2 = make(map[string]int) //자료형 생략
map3 := make(map[string]int) //리터럴 형
fmt.Println("ex1 : ", map1)
fmt.Println("ex1 : ", map2)
fmt.Println("ex1 : ", map3)
fmt.Println()
//예제2
map4 := map[string]int{}
map4["apple"] = 25
map4["banana"] = 40
map4["orange"] = 33
map5 := map[string]int{
"apple": 15,
"banana": 30,
"orange": 23, //콤마 주의
}
map6 := make(map[string]int, 10)
map6["apple"] = 5
map6["banana"] = 10
map6["orange"] = 15
fmt.Println("ex2 : ", map4)
fmt.Println("ex2 : ", map5)
fmt.Println("ex2 : ", map6)
fmt.Println("ex2 : ", map6["orange"])
fmt.Println("ex2 : ", map6["apple"])
fmt.Println()
}
package main
import "fmt"
func main() {
//맵(Map)
//맵 조회 및 순회(Iterator)
//예제1
map1 := map[string]string{
"daum": "http://daum.net",
"naver": "http://naver.com",
"google": "http://google.com",
}
fmt.Println("ex1 : ", map1["google"])
fmt.Println("ex1 : ", map1["daum"])
fmt.Println()
//예제2(순서 없으므로 랜덤)
for k, v := range map1 {
fmt.Println("ex2 : ", k, v)
}
fmt.Println()
for _, v := range map1 { //변수 선언 후 사용안하면 예외 발생
fmt.Println("ex2 : ", v)
}
}
package main
import "fmt"
func main() {
//맵(Map)
//맵 값 변경 및 삭제
//예제1(수정)
map1 := map[string]string{
"daum": "http://daum.net",
"naver": "http://naver.com",
"google": "http://google.com",
"home1": "http://test1.com",
}
fmt.Println("ex1 : ", map1)
map1["home2"] = "http://test2.com" //추가
fmt.Println("ex1 : ", map1)
map1["home2"] = "http://test2-2.com" //수정
fmt.Println("ex1 : ", map1)
fmt.Println()
//예제2(삭제)
delete(map1, "home2")
fmt.Println("ex1 : ", map1)
delete(map1, "home1")
fmt.Println("ex1 : ", map1)
}
package main
import "fmt"
func main() {
//맵(Map)
//맵 조회 할 경우 주의 할점
//예제1
map1 := map[string]int{
"apple": 15,
"banana": 115,
"orange": 1115,
"lemon": 0,
}
value1 := map1["lemon"]
value2 := map1["kiwi"]
value3, ok := map1["kiwi"]
fmt.Println("ex1 : ", value1)
fmt.Println("ex1 : ", value2)
fmt.Println("ex1 : ", value3, ok) //두 번째 리턴 값으로 키 존재 유무 확인
fmt.Println()
//예제2
if value, ok := map1["kiwi"]; ok {
fmt.Println("ex2 : ", value)
} else {
fmt.Println("ex2 : kiwi is not exist!")
}
if value, ok := map1["lemon"]; ok {
fmt.Println("ex2 : ", value)
} else {
fmt.Println("ex2 : kiwi is not exist!")
}
if _, ok := map1["kiwi"]; !ok {
fmt.Println("ex2 : kiwi is not exist!")
}
}