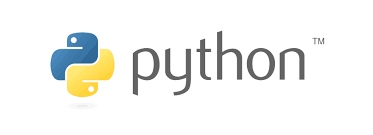
print(10 + 5)
print(10 - 5)
print(10 * 5)
print(10 / 5)
import math
print(math.ceil(2.2))
print(math.floor(2.7))
print(math.pow(2,10))
print(math.pi)
print('Hello')
print("Hello")
print("Hello 'world'")
print('Hello "world"')
# 실행결과
$ Hello
$ Hello
$ Hello 'world'
$ Hello "world"
print('Hello '+'world')
print('Hello '*3)
print('Hello'[0])
print('Hello'[1])
print('Hello'[2])
# 실행결과
$ Hello world
$ Hello Hello Hello
$ H
$ e
$ l
print('hello world'.capitalize())
print('hello world'.upper())
print('hello world'.__len__())
print(len('hello world'))
print('Hello world'.replace('world', 'programming'))
# 실행결과
$ Hello world
$ HELLO WORLD
$ 11
$ 11
$ Hello programming
변수
name = "이상효"
print("안녕하세요. "+name+"님")
print(name+"님을 위한 강의를 준비했습니다.")
print(name+"님 꼭 참석 부탁드립니다.")
donation = 200
student = 10
sponsor = 100
print((donation*student)/sponsor)
if
input = 33
real_egoing = 11
real_k8805 = "ab"
if real_egoing == input:
print("Hello!, egoing")
elif real_k8805 == input:
print("Hello!, k8805")
else:
print("Who are you?")
in_str = input("아이디를 입력해주세요.\n")
real_egoing = "egoing"
real_k8805 = "k8805"
if real_egoing == in_str or real_k8805 == in_str:
print("Hello!")
else:
print("Who are you?")
주석
'''
조건문 예제
egoing
2015
'''
"""
이거도 됨.
"""
# user input password
input = 33
real_egoing = 11
#real_k8805 = "ab"
if real_egoing == input:
print("Hello!, egoing")
#elif real_k8805 == input:
# print("Hello!, k8805")
else:
print("Who are you?")
list 데이터 타입
print(type('egoing')) #<class 'str'>
name = 'egoing'
print(name) #egoing
print(type(['egoing', 'leezche', 'graphittie'])) #<class 'list'>
names = ['egoing', 'leezche', 'graphittie']
print(names)
print(names[2]) #graphittie
egoing = ['programmer', 'seoul', 25, False]
egoing[1] = 'busan'
print(egoing) #['programmer', 'busan', 25, False]
반복문
i = 0
while i < 3:
print('Hello world')
i = i + 1
i = 0
while i < 10:
print('print("Hello world '+str(i*9)+'")')
i = i + 1
i = 0
while i < 10:
if i == 4:
break
print(i)
i = i + 1
print('after while')
input_id = input("아이디를 입력해주세요.\n")
members = ['egoing', 'k8805', 'leezche']
for member in members:
if member == input_id:
print('Hello!, '+member)
import sys
sys.exit()
print('Who are you?')
함수
def a3():
print('aaa')
a3()
def a3():
return 'aaa'
print(a3())
def a(num):
return 'a'*num
print(a(3))
def make_string(str, num):
return str*num
print(make_string('b', 3))
input_id = input("아이디를 입력해주세요.\n")
def login(_id):
members = ['egoing', 'k8805', 'leezche']
for member in members:
if member == _id:
return True
return False
if login(input_id):
print('Hello, '+input_id)
else:
print('Who are you?')
모듈(외부 함수) 사용하기
python 내장 모듈
import math
print(math.ceil(2.9))
print(math.floor(2.9))
print(math.sqrt(16))
# 실행결과
3
2
4.0
모듈 작성
# egoing.py
def a():
return 'a'
def b():
return 'b'
def c():
return 'c'
# k8805.py
def a():
return 'B'
from egoing import a as z
import k8805 as k
print(z())
print(k.a())
모듈 작성2
# auth.py
def login(_id):
members = ['egoing', 'k8805', 'leezche']
for member in members:
if member == _id:
return True
return False
import auth
input_id = input("아이디를 입력해주세요.\n")
if auth.login(input_id):
print('Hello, '+input_id)
else:
print('Who are you?')
클래스
class Cal(object):
def __init__(self, v1, v2):
self.v1 = v1
self.v2 = v2
def add(self):
return self.v1+self.v2
def subtract(self):
return self.v1-self.v2
c1 = Cal(10,10)
print(c1.add())
print(c1.subtract())
c2 = Cal(30,20)
print(c2.add())
print(c2.subtract())
상속
class Class1(object):
def method1(self): return 'm1'
c1 = Class1()
print(c1, c1.method1())
class Class3(Class1):
def method2(self): return 'm2'
c3 = Class3()
print(c3, c3.method1())
print(c3, c3.method2())
class Class2(object):
def method1(self): return 'm1'
def method2(self): return 'm2'
c2 = Class2()
print(c2, c2.method1())
print(c2, c2.method2())
static, class 선언
class Cs:
@staticmethod
def static_method():
print("Static method")
@classmethod
def class_method(cls):
print("Class method")
def instance_method(self):
print("Instance method")
i = Cs()
Cs.static_method()
Cs.class_method()
i.instance_method()
class Cs:
count = 0
def __init__(self):
Cs.count = Cs.count + 1
@classmethod
def getCount(cls):
return Cs.count
i1 = Cs()
i2 = Cs()
i3 = Cs()
i4 = Cs()
print(Cs.getCount())