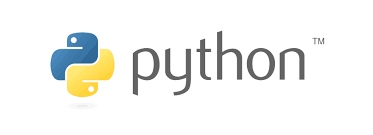
디폴트 install, import
!pip install finance-datareader
!pip install beautifulsoup4
!pip install numpy
!pip install pandas
# default settings
import numpy as np
import pandas as pd
from datetime import datetime
# jupyter notebook 여러 실행인자 실행해도 print되게 만들기
from IPython.core.interactiveshell import InteractiveShell
InteractiveShell.ast_node_interactivity = "all"
pd.set_option('display.float_format', lambda x: '%.3f' % x)
pd.set_option('max_columns', None)
5. datetime 시작
timestamp 써보기
선언은 이렇게 할 수 있다
datetime(2021, 1, 1)
type(datetime(2021, 1, 1))
datetime.datetime(2021, 1, 1, 0, 0)
datetime.datetime
a = datetime(2014, 8, 1)
b = pd.Timestamp(a)
b
Timestamp('2014-08-01 00:00:00')
pd.Timestamp("2021-01-02")
Timestamp('2021-01-02 00:00:00')
datetime은 주로 index로 사용이 된다.
dates = [datetime(2014, 8, 1), datetime(2014, 8, 5)]
type(dates)
list
dti = pd.DatetimeIndex(dates)
dti
DatetimeIndex(['2014-08-01', '2014-08-05'], dtype='datetime64[ns]', freq=None)
pd.to_datetime(dates)
DatetimeIndex(['2014-08-01', '2014-08-05'], dtype='datetime64[ns]', freq=None)
pd.to_datetime(dates)[0]
Timestamp('2014-08-01 00:00:00')
Series와 함께 사용되는 예제를 보자
dates = [datetime(2014, 8, 1), datetime(2014, 8, 5)]
ts = pd.Series(np.random.randn(2), index=dates)
ts
2014-08-01 0.181
2014-08-05 -0.232
dtype: float64
ts.index
DatetimeIndex(['2014-08-01', '2014-08-05'], dtype='datetime64[ns]', freq=None)
ts.loc[pd.Timestamp("2014-08-01")]
0.18080412810914337
ts.loc[datetime(2014, 8, 1)]
ts.loc["2014-08-01"]
0.18080412810914337
0.18080412810914337
주의사항
# True
pd.Timestamp(dates[0]) == datetime(2014, 8, 1)
pd.to_datetime(dates)[0] == datetime(2014, 8, 1)
# False
pd.to_datetime(dates)[0] == "2014-08-01"
True
True
False
# sorting도 지원
ts = ts.sort_index()
ts
2014-08-01 0.181
2014-08-05 -0.232
dtype: float64
ts.loc["2014-08-01"]
0.18080412810914337
ts.loc["2014-08"]
2014-08-01 0.181
2014-08-05 -0.232
dtype: float64
ts.loc["2014-08-01":]
2014-08-01 0.181
2014-08-05 -0.232
dtype: float64
# 주의! list 인덱싱과는 다르게 양끝 포함
ts.loc["2014-08-01":"2014-08-05"]
2014-08-01 0.181
2014-08-05 -0.232
dtype: float64
주기적으로 반복되는 datetime도 넣을 수 있음.
dates = pd.date_range('2014-08-01', periods=10, freq="D")
dates
DatetimeIndex(['2014-08-01', '2014-08-02', '2014-08-03', '2014-08-04',
'2014-08-05', '2014-08-06', '2014-08-07', '2014-08-08',
'2014-08-09', '2014-08-10'],
dtype='datetime64[ns]', freq='D')
dates = pd.date_range('2014-08-01', periods=10, freq="B")
dates
DatetimeIndex(['2014-08-01', '2014-08-04', '2014-08-05', '2014-08-06',
'2014-08-07', '2014-08-08', '2014-08-11', '2014-08-12',
'2014-08-13', '2014-08-14'],
dtype='datetime64[ns]', freq='B')
dates = pd.date_range('2014-08-01', "2014-08-14", freq="D")
dates
DatetimeIndex(['2014-08-01', '2014-08-02', '2014-08-03', '2014-08-04',
'2014-08-05', '2014-08-06', '2014-08-07', '2014-08-08',
'2014-08-09', '2014-08-10', '2014-08-11', '2014-08-12',
'2014-08-13', '2014-08-14'],
dtype='datetime64[ns]', freq='D')
주기가 길어질 경우 아래와 같이 처리해 보자
period = pd.Period('2014-08', freq='Q') # freq= "D", "M", .. etc
period
Period('2014Q3', 'Q-DEC')
period.start_time
period.end_time
Timestamp('2014-07-01 00:00:00')
Timestamp('2014-09-30 23:59:59.999999999')
# +1 ==> `freq`에 해당하는 단위가 더해짐 (여기서는 1Q)
period2 = period + 1
period2
Period('2014Q4', 'Q-DEC')
period2.start_time
period2.end_time
Timestamp('2014-10-01 00:00:00')
Timestamp('2014-12-31 23:59:59.999999999')
pandas에는 period_range라는 함수도 지원한다
p2013 = pd.period_range('2013-01-01', '2013-12-31', freq='M')
p2013
PeriodIndex(['2013-01', '2013-02', '2013-03', '2013-04', '2013-05', '2013-06',
'2013-07', '2013-08', '2013-09', '2013-10', '2013-11', '2013-12'],
dtype='period[M]')
p2013[0]
Period('2013-01', 'M')
for p in p2013:
print("{0} {1} {2} {3}".format(p, p.freq, p.start_time, p.end_time))
2013-01 <MonthEnd> 2013-01-01 00:00:00 2013-01-31 23:59:59.999999999
2013-02 <MonthEnd> 2013-02-01 00:00:00 2013-02-28 23:59:59.999999999
2013-03 <MonthEnd> 2013-03-01 00:00:00 2013-03-31 23:59:59.999999999
2013-04 <MonthEnd> 2013-04-01 00:00:00 2013-04-30 23:59:59.999999999
2013-05 <MonthEnd> 2013-05-01 00:00:00 2013-05-31 23:59:59.999999999
2013-06 <MonthEnd> 2013-06-01 00:00:00 2013-06-30 23:59:59.999999999
2013-07 <MonthEnd> 2013-07-01 00:00:00 2013-07-31 23:59:59.999999999
2013-08 <MonthEnd> 2013-08-01 00:00:00 2013-08-31 23:59:59.999999999
2013-09 <MonthEnd> 2013-09-01 00:00:00 2013-09-30 23:59:59.999999999
2013-10 <MonthEnd> 2013-10-01 00:00:00 2013-10-31 23:59:59.999999999
2013-11 <MonthEnd> 2013-11-01 00:00:00 2013-11-30 23:59:59.999999999
2013-12 <MonthEnd> 2013-12-01 00:00:00 2013-12-31 23:59:59.999999999
# DateTimeIndex : collections of `Timestamp` objects
a = pd.date_range('1/1/2013', '12/31/2013', freq='M')
a
a[0]
DatetimeIndex(['2013-01-31', '2013-02-28', '2013-03-31', '2013-04-30',
'2013-05-31', '2013-06-30', '2013-07-31', '2013-08-31',
'2013-09-30', '2013-10-31', '2013-11-30', '2013-12-31'],
dtype='datetime64[ns]', freq='M')
Timestamp('2013-01-31 00:00:00', freq='M')
# PeriodIndex : collections of `Period` objects
b = pd.period_range('1/1/2013', '12/31/2013', freq='M')
b
b[0]
PeriodIndex(['2013-01', '2013-02', '2013-03', '2013-04', '2013-05', '2013-06',
'2013-07', '2013-08', '2013-09', '2013-10', '2013-11', '2013-12'],
dtype='period[M]')
Period('2013-01', 'M')
# 해당 index는 이제 특정 date(time) 시점을 의미하는 것이 아닌, date 범위(range)를 의미
ps = pd.Series(np.random.randn(12), p2013)
ps
2013-01 -0.083
2013-02 1.099
2013-03 0.049
2013-04 1.564
2013-05 -1.250
2013-06 -0.928
2013-07 -0.158
2013-08 1.048
2013-09 -0.387
2013-10 -0.322
2013-11 -0.925
2013-12 -0.483
Freq: M, dtype: float64
ps.loc["2013-11"]
-0.9245662902064992
ps.loc["2013-11":]
2013-11 -0.925
2013-12 -0.483
Freq: M, dtype: float64