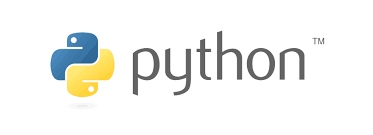
# 기본 람다 사용법
plus_ten = lambda x : x + 10
print(plus_ten(1))
# 람다식 + 쓰레드
threading.Thread(target=lambda a: print("Hello, {}".format(a)), args=(["world"])).start()
# or
new_thread = threading.Thread(target=lambda a: print("Hello, {}".format(a)), args=(["world"]))
new_thread.start()
# 람다식 + 쓰레드 + 변수사용
a = "Hell Wrold!"
new_thread = threading.Thread(target=lambda : print("Hello, {}".format(a)))
new_thread.start()
def sum(low, high):
total = 0
for i in range(low, high):
total += i
print("Subthread", total)
t = threading.Thread(target=sum, args=(1, 10000))
t.daemon = True # 데몬쓰레드 설정(메인쓰레드와 생명주기를 같이한다)
t.start()
async def async_fun():
print("Hello Async")
# 기본 async 사용법
loop = asyncio.get_event_loop()
loop.run_until_complete(async_fun())
loop.close()
# python 3.7 이상에선 아래 명령으로 처리
# asyncio.run(async_fun())
# 아래를 비동기로 처리해보자
def find_users_sync(n):
for i in range(1, n + 1):
time.sleep(1)
print(f"--> 총 {n} 명 사용자 조회 완료!")
def sync_func():
start = time.time()
find_users_sync(3)
find_users_sync(2)
find_users_sync(1)
end = time.time()
print(f"--> 총 소요 시간 {end - start}")
sync_func()
async def find_users_async(n):
for i in range(1, n + 1):
time.sleep(1)
print(f"--> 총 {n} 명 사용자 조회 완료!")
async def async_func():
start = time.time()
await asyncio.wait([
find_users_async(3),
find_users_async(2),
find_users_async(1),
])
end = time.time()
print(f"--> 총 소요 시간 {end - start}")
loop = asyncio.get_event_loop()
loop.run_until_complete(async_func())
loop.close()
# Python 3.7 이상 표현
# asyncio.run(async_func())