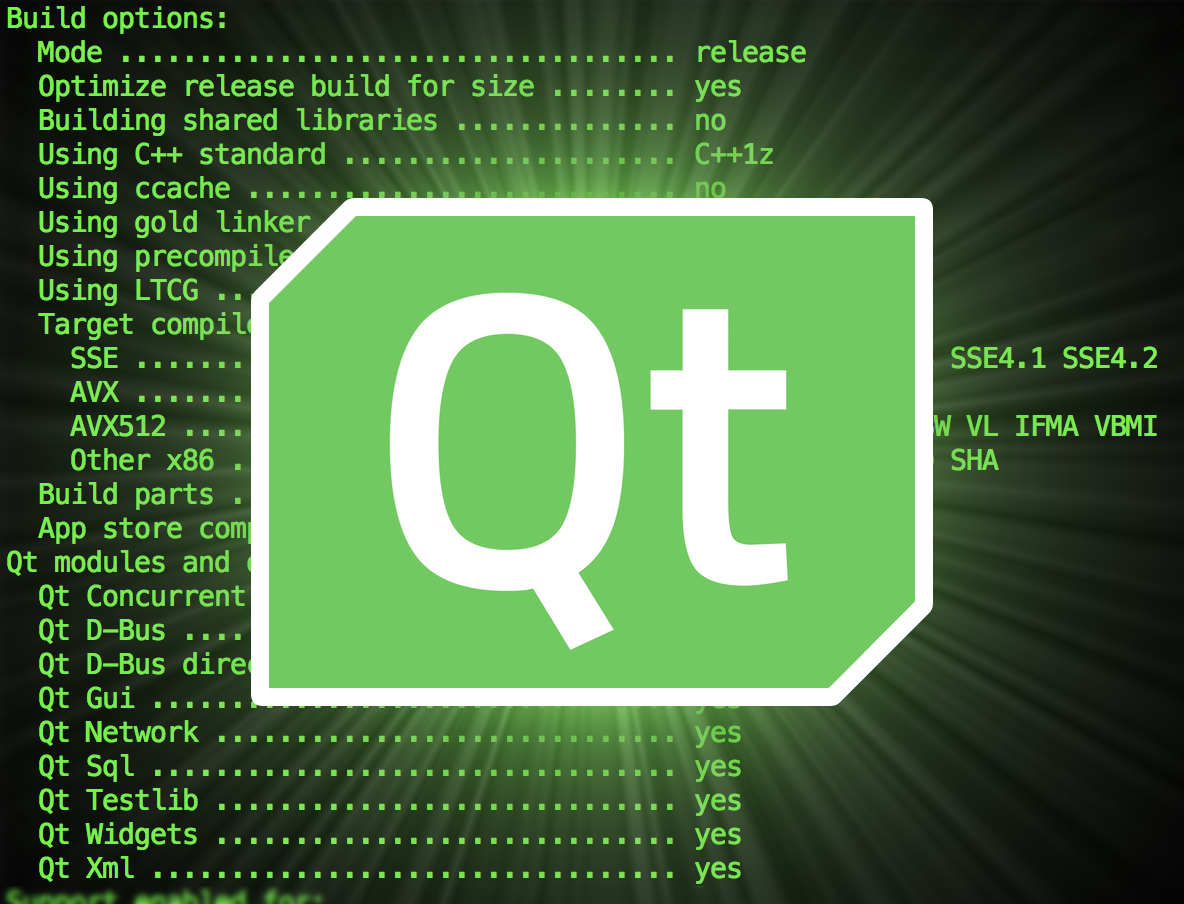
singleShot
#include <QCoreApplication>
#include <QTimer>
void test()
{
qInfo() << "Thank you for waiting!";
}
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
qInfo() << "Please wait...";
QTimer::singleShot(5000,&test);
return a.exec();
}
inserval
#include "test.h"
Test::Test(QObject *parent) : QObject(parent)
{
number = 0;
timer.setInterval(1000);
connect(&timer,&QTimer::timeout,this,&Test::timeout);
}
void Test::timeout()
{
number++;
//Qt5
//qInfo() << QTime::currentTime().toString(Qt::DateFormat::SystemLocaleLongDate);
qInfo() << QTime::currentTime().toString(Qt::DateFormat::TextDate);
if(number >= 5)
{
timer.stop();
qInfo() << "Complete!";
}
}
void Test::dostuff()
{
number = 0;
timer.start();
}
Process timeout
#include <QCoreApplication>
#include <QProcess>
#include <QTimer>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
QProcess proc;
proc.start("xed");
QTimer::singleShot(3000,&proc,&QProcess::terminate);
return a.exec();
}