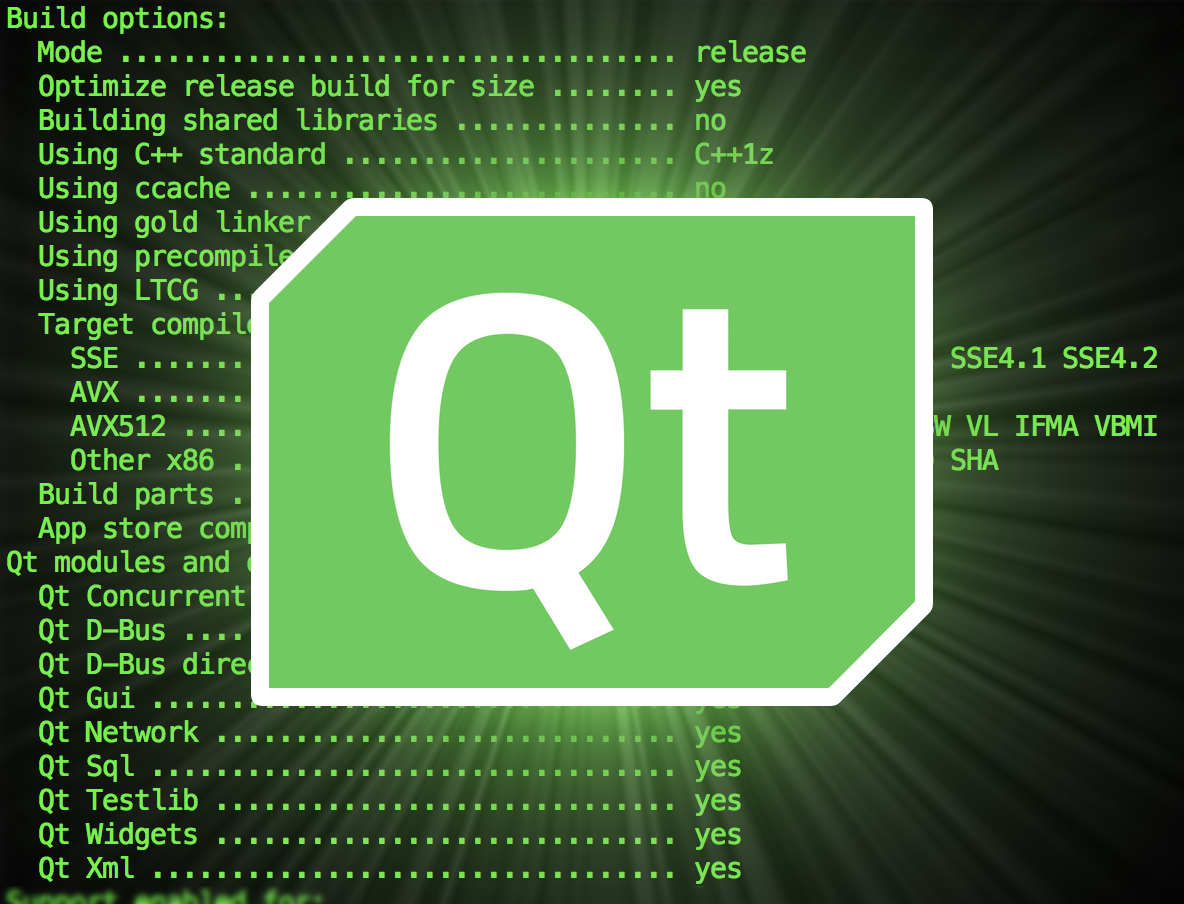
Main QThread 실행확인
#include <QCoreApplication>
#include <QThread>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
QThread *thread = QThread::currentThread();
thread->setObjectName("Main Thread");
qInfo() << "Starting" << thread;
for(int i = 0; i < 10; i++)
{
qInfo() << i << "on" << thread;
}
qInfo() << "Finished" << thread;
return a.exec();
}
QObject QThread에서 실행해 보기
// Task.h
#ifndef TASK_H
#define TASK_H
#include <QObject>
#include <QThread>
#include <QDebug>
class Task : public QObject
{
Q_OBJECT
public:
explicit Task(QObject *parent = nullptr);
~Task();
signals:
public slots:
void work();
};
#endif // TASK_H
#include "task.h"
Task::Task(QObject *parent) : QObject(parent)
{
qInfo() << "Constructed" << this << "on" << QThread::currentThread();
}
Task::~Task()
{
qInfo() << "Deconstructed" << this << "on" << QThread::currentThread();
}
void Task::work()
{
QThread *thread = QThread::currentThread();
qInfo() << "Starting" << thread;
for(int i = 0; i < 10; i++)
{
qInfo() << i << "on" << thread;
}
qInfo() << "Finished" << thread;
}
#include <QCoreApplication>
#include <QThread>
#include <QDebug>
#include <QScopedPointer>
#include "task.h"
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
QThread::currentThread()->setObjectName("Main Thread");
QThread worker;
worker.setObjectName("Worker Thread");
qInfo() << "Starting work" << QThread::currentThread();
//Task *task = new Task(&a);
QScopedPointer<Task> t(new Task()); //Auto delete
Task *task = t.data();
task->moveToThread(&worker);
worker.connect(&worker,&QThread::started, task,&Task::work);
//Connect to QThread::finished if you need to know when the thread is done
worker.start();
qInfo() << "Free to do other things..." << QThread::currentThread();
return a.exec();
}