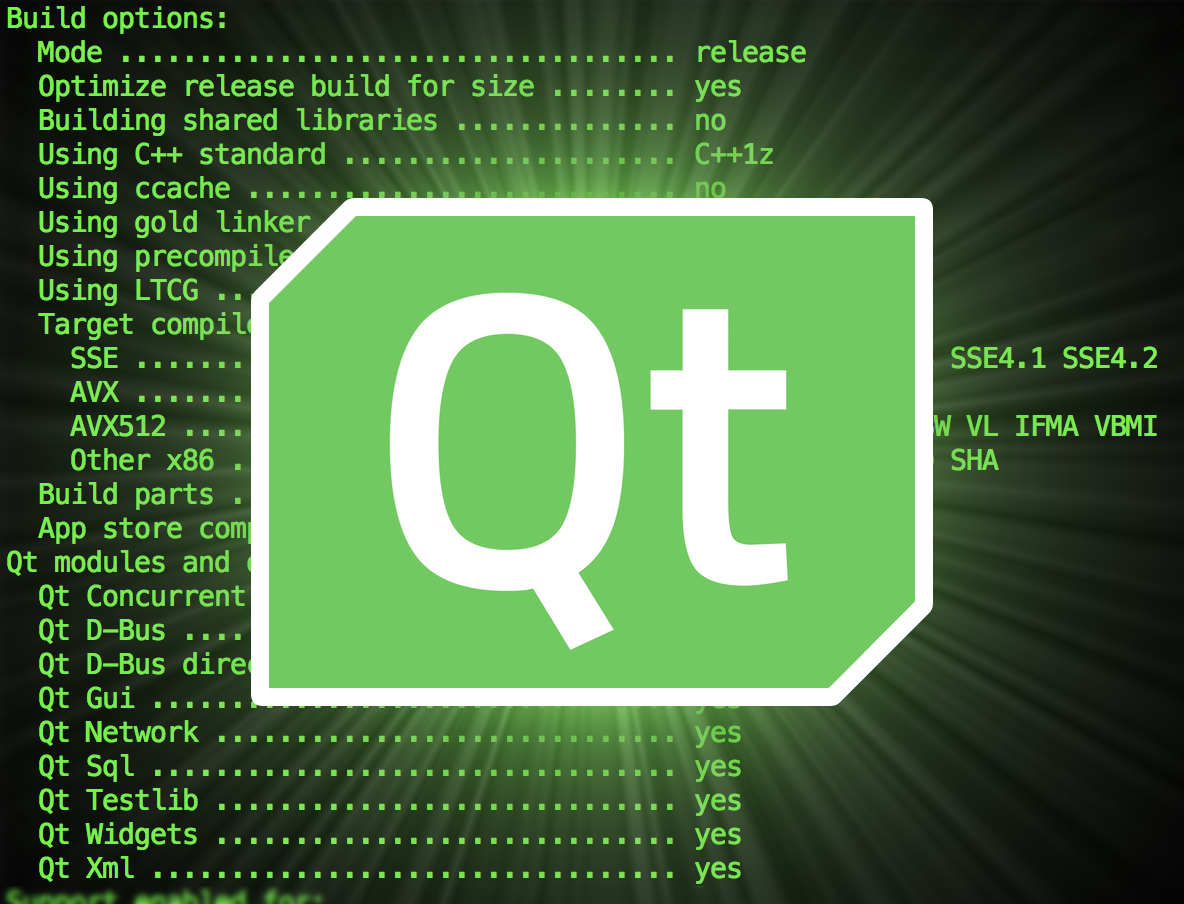
- We want multiple resuable threads
- QTreadPool and QRunnable
// main
#include <QCoreApplication>
#include <QDebug>
#include <QThread>
#include <QThreadPool>
#include "counter.h"
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
QThread::currentThread()->setObjectName("Main");
QThreadPool* pool = QThreadPool::globalInstance();
qInfo() << pool->maxThreadCount() << "Threads";
for(int i = 0; i < 100; i++)
{
Counter* c = new Counter();
c->setAutoDelete(true);
pool->start(c);
}
return a.exec();
}
// counter.h
#ifndef COUNTER_H
#define COUNTER_H
#include <QObject>
#include <QDebug>
#include <QRunnable>
#include <QThread>
#include <QRandomGenerator>
class Counter : public QRunnable
{
public:
Counter();
// QRunnable interface
public:
void run();
};
#endif // COUNTER_H
// counter.cpp
#include "counter.h"
Counter::Counter()
{
}
void Counter::run()
{
qInfo() << "Starting: " << QThread::currentThread();
for (int i = 0; i < 20; i++) {
//comment this out to see the threads being reused
qInfo() << QThread::currentThread() << " = " << i;
auto value = static_cast<unsigned long>(QRandomGenerator::global()->bounded(100));
QThread::currentThread()->msleep(value);
}
qInfo() << "Finished: " << QThread::currentThread();
}