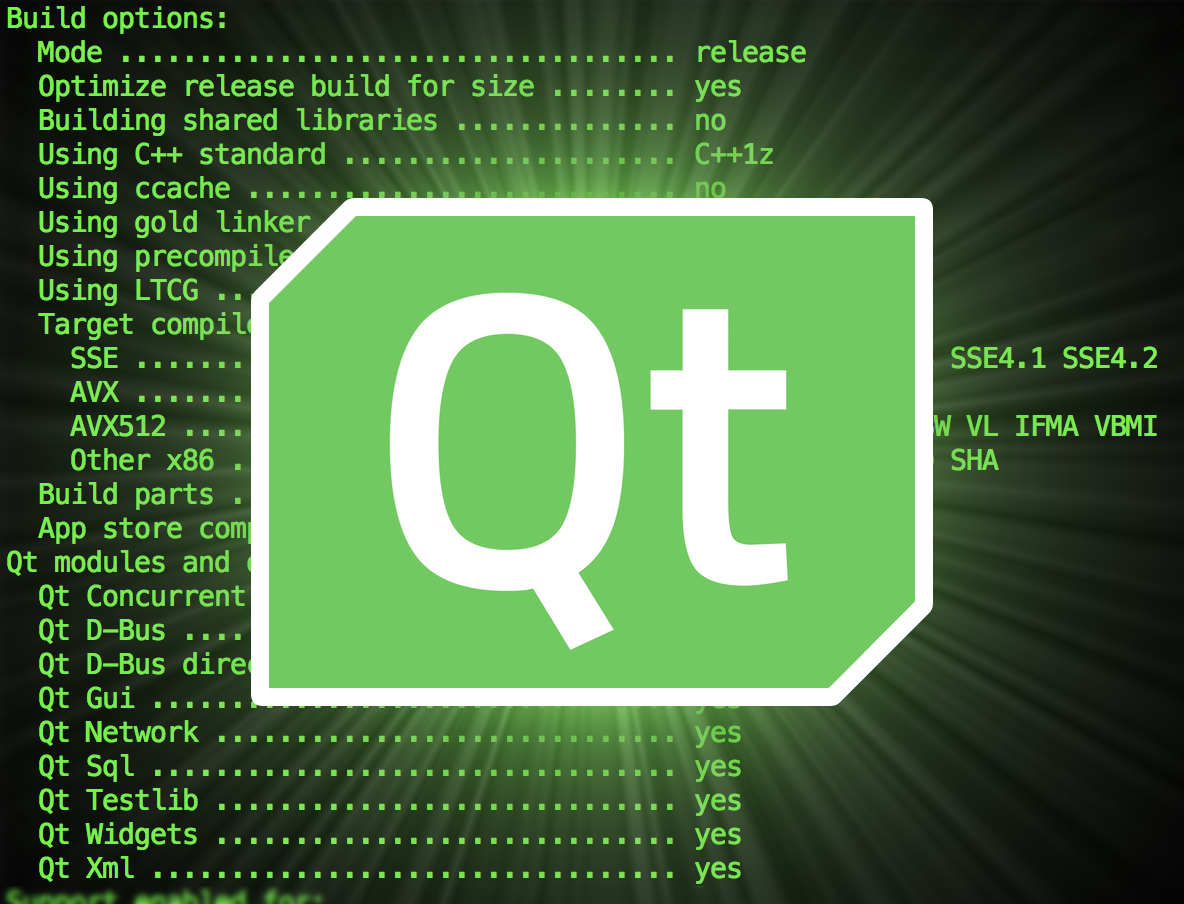
Producer::Producer(QMutex *mutex, QWaitCondition *valueReady, int *value, QObject *parent)
{
Q_UNUSED(parent);
m_mutex = mutex;
m_valueReady = valueReady;
m_value = value;
}
void Producer::run()
{
qInfo() << "Producer running";
QThread::currentThread()->msleep(3000);
m_mutex->lock();
qInfo() << "Producer creating value";
*m_value = QRandomGenerator::global()->bounded(1000);
qInfo() << "Producer waking up consumer";
m_mutex->unlock();
// wait 중인 WaitCondition을 깨운다
m_valueReady->wakeAll();
}
Consumer::Consumer(QMutex *mutex, QWaitCondition *valueReady, int *value, QObject *parent)
{
Q_UNUSED(parent);
m_mutex = mutex;
m_valueReady = valueReady;
m_value = value;
}
void Consumer::run()
{
qInfo() << "Consumer running";
m_mutex->lock();
qInfo() << "Consumer locked the mutex";
if(*m_value == 0)
{
qInfo() << "Consumer is waiting on a value";
m_valueReady->wait(m_mutex);
}
qInfo() << "Consuming:" << *m_value;
}