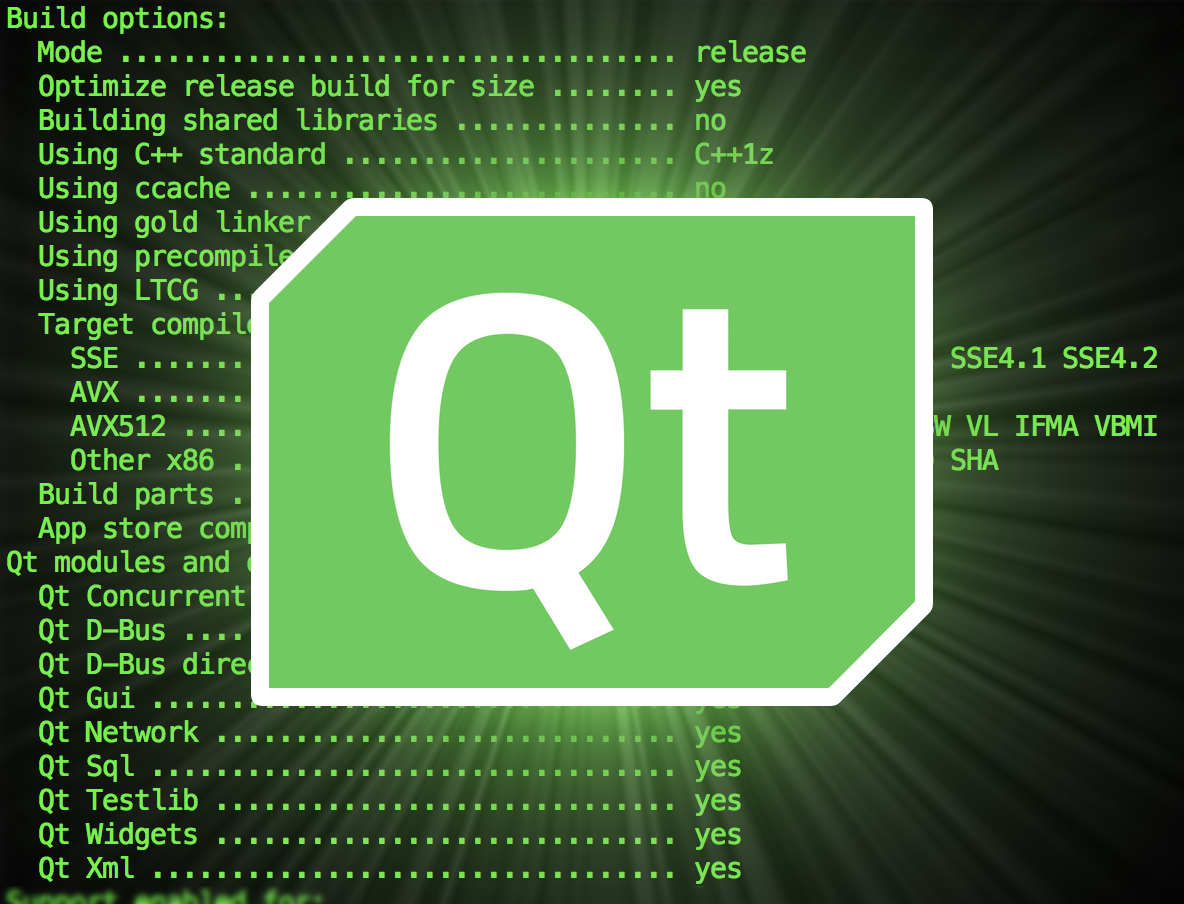
#include <QCoreApplication>
#include <QTest>
#include "cat.h"
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
Cat cat;
#ifdef QT_DEBUG
QTest::qExec(&cat);
#endif
return a.exec();
}
#ifndef CAT_H
#define CAT_H
#include <QObject>
#include <QDebug>
class Cat : public QObject
{
Q_OBJECT
public:
explicit Cat(QObject *parent = nullptr);
void public_test(); //not called
signals:
public slots:
void test(); //not called
private slots:
void play();
void meow();
void sleep();
void speak(QString value); //no param
private:
void private_test(); //not called
};
#endif // CAT_H
WARN, ERROR
Widget::Widget(QObject *parent) : QObject(parent)
{
age = 0;
}
void Widget::setAge(int value)
{
age = value;
}
void Widget::testFail()
{
QFAIL("NO REASON JUST FAIL!!!");
}
void Widget::testAge()
{
if(!age) QFAIL("Age is not set!");
}
void Widget::testWidget()
{
int value = 45;
//Make sure the age is valid--------------
QVERIFY(age > 0 && age < 100);
//Issue warnings
if(age > 40) QWARN("Age is over 40!");
if(age < 21) QFAIL("Must be an adult!");
//Make sure they are the same---------------------
QCOMPARE(age, value);
}
void Widget::testAge_data()
{
qInfo() << "Generating data...";
QTest::addColumn<QString>("name");
QTest::addColumn<int>("age");
QTest::addRow("Invalid") << "Bob" << 190;
QTest::addRow("Old") << "Bryan" << 44;
QTest::addRow("Young") << "Heather" << 25;
QTest::addRow("Under age") << "Rango" << 14;
QTest::addRow("Retired") << "Grandma" << 90;
qInfo() << "Data generated!";
}
void Widget::testAge()
{
QFETCH(QString,name);
QFETCH(int, age);
qInfo() << "Testing age " << name << " is " << age;
if(age < 1 || age > 100) QFAIL("Invalid Age!");
if(age < 21) QFAIL("Must be an adult!");
if(age > 40) QWARN("Getting Old!");
if(age > 65) qInfo() << "This person is retired";
}
BENCHMARK CHECK
#include "widget.h"
Widget::Widget(QObject *parent) : QObject(parent)
{
}
void Widget::testFor()
{
QVector<int> list;
list.fill(0,100);
//Called multiple times!
QBENCHMARK
{
for (int i = 0; i < list.size();i++)
{
//Do Stuff
}
}
}
void Widget::testForEach()
{
QVector<int> list;
list.fill(0,100);
//Called multiple times!
QBENCHMARK
{
foreach(int value, list)
{
//Do Stuff
}
}
}
void Widget::testString()
{
QString him = "Bryan";
QString her = "Tammy";
QBENCHMARK
{
int ret = him.compare(her);
}
}
void Widget::testComp()
{
QString him = "Bryan";
QString her = "Tammy";
QBENCHMARK
{
QCOMPARE(him, her);
}
}