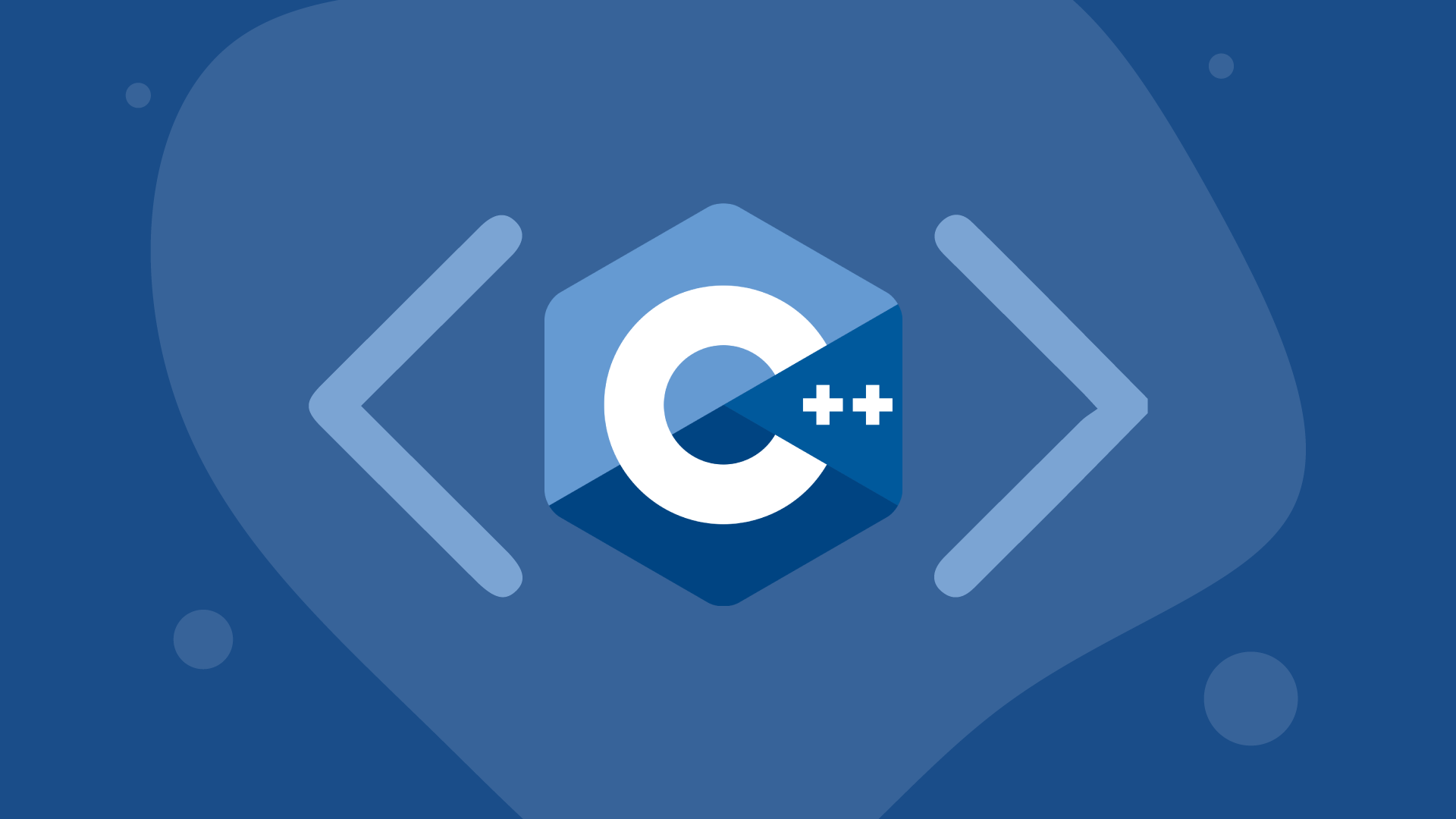
mutable
class Point
{
int x, y;
// mutable 선언
mutable int cnt = 0;
public:
Point(int a = 0, int b = 0) : x(a), y(b) {}
void print() const
{
++ cnt; // 상수함수인데 private 변수 변경가능.
std::cout << x << ", " << y << std::endl;
}
};
Example
#include <iostream>
using namespace std;
int g = 10;
int main()
{
int v1 = 0, v2 = 0;
auto f1 = [](int a) { return a + g; }
// 람다표현식에서 전역변수에 접근이 가능? -> Okay, 읽고, 쓰고 모두 가능
auto f2 = [](int a) { return a + v1; }
// error - 지역변수 접근에는 에러가 난다.
auto f3 = [v1](int a) { return a + v1; }
// 지역변수 캡처를 지정해 줘야한다.
auto f4 = [v1](int a) mutable { v1 = 10; }
// 값 캡쳐인데
// mutable을 쓰면 값 변경도 가능!
}
// 지역변수 캡처시 값변경은?
auto f1 = [v1, v2]() {v1 = 10; v2 = 20; }
// error! -> operator()() const 상수이기에 변경 불가!
// mutable lambda : ()연산자 함수를 비상수 함수로
auto f1 = [v1, v2]() mutable {v1 = 10; v2 = 20; }
f1();
cout << v1 << endl; // 0
cout << v2 << endl; // 0
// 복사본을 변경했기에 원본은 변화가 없음.