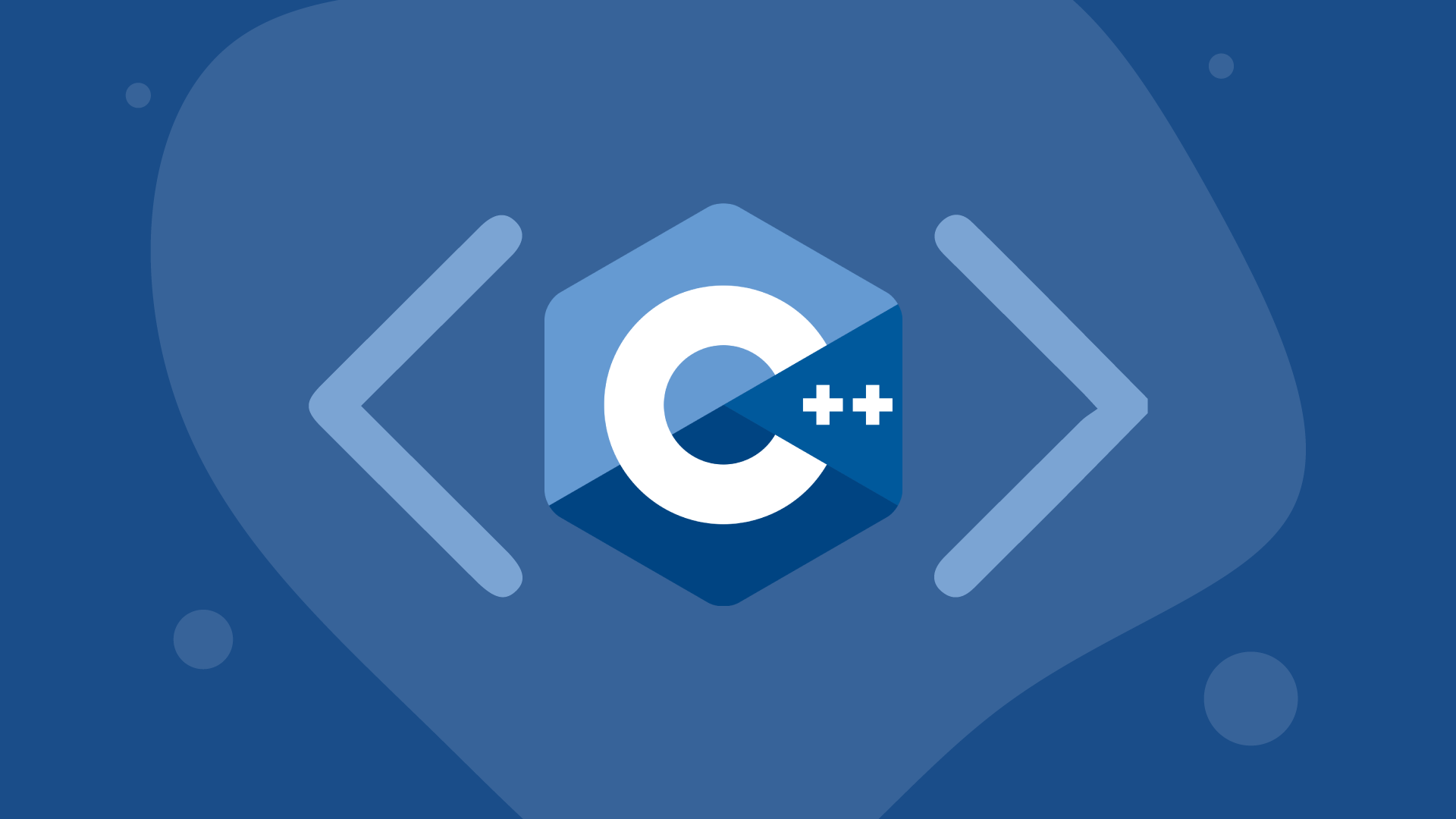
std::this_thread
std::this_thread::get_id()
#include <iostream>
#include <thread>
int main()
{
// 현 thread의 id값을 반환
std::cout << std::this_thread::get_id() << std::endl;
std::thread::id tid1 = std::this_thread::get_id();
std::thread::id tid2 = std::this_thread::get_id();
// std::this_thread::get_id()의 리턴이 int값이 아니라 std::thead::id라는 값
// 아래와 같이 비교연산도 가능
// 단, 정수변환은 불가능!
tid1 == tid2;
tid1 < tid2;
// key값으로 사용이 가능
std::hash<std::thread:id> h;
std::cout << h(tid1) << std::endl;
}
sleep()
#include <iostream>
#include <thread>
#include <chrono>
using namespace std::literals;
int main()
{
// sleep_for() : 이 시간 동안 멈춰주세요
std::this_thread::sleep_for(std::chrono::seconds(3));
std::this_thread::sleep_for(3s);
std::this_thread::sleep_for(3);
// sleep_until() : 이 시간 까지 멈춰주세요
std::chrono::time_point tp1 = std::chrono::steady_clock::now(); // 현재시간
std::this_thread::sleep_until(tp1 + 2000ms);
auto tp2 = createDataTime(2021, 4, 11, 12, 39, 00); // 특정날자에 깨우려면
std::this_thread::sleep_until(tp2);
}
yield()
#include <iostream>
#include <thread>
#include <chrono>
using namespace std::literals;
void mysleep(std::chrono::microseconds us)
{
auto target = std::chrono::high_resolution_clock::now() + us;
while(std::chrono::high_resolution_clock::now() < target)
std::this_thread::yield();
// 현재 스레드의 실행흐름을 다른 스레드에게 넘긴다
}
int main()
{
mysleep(10ms);
}