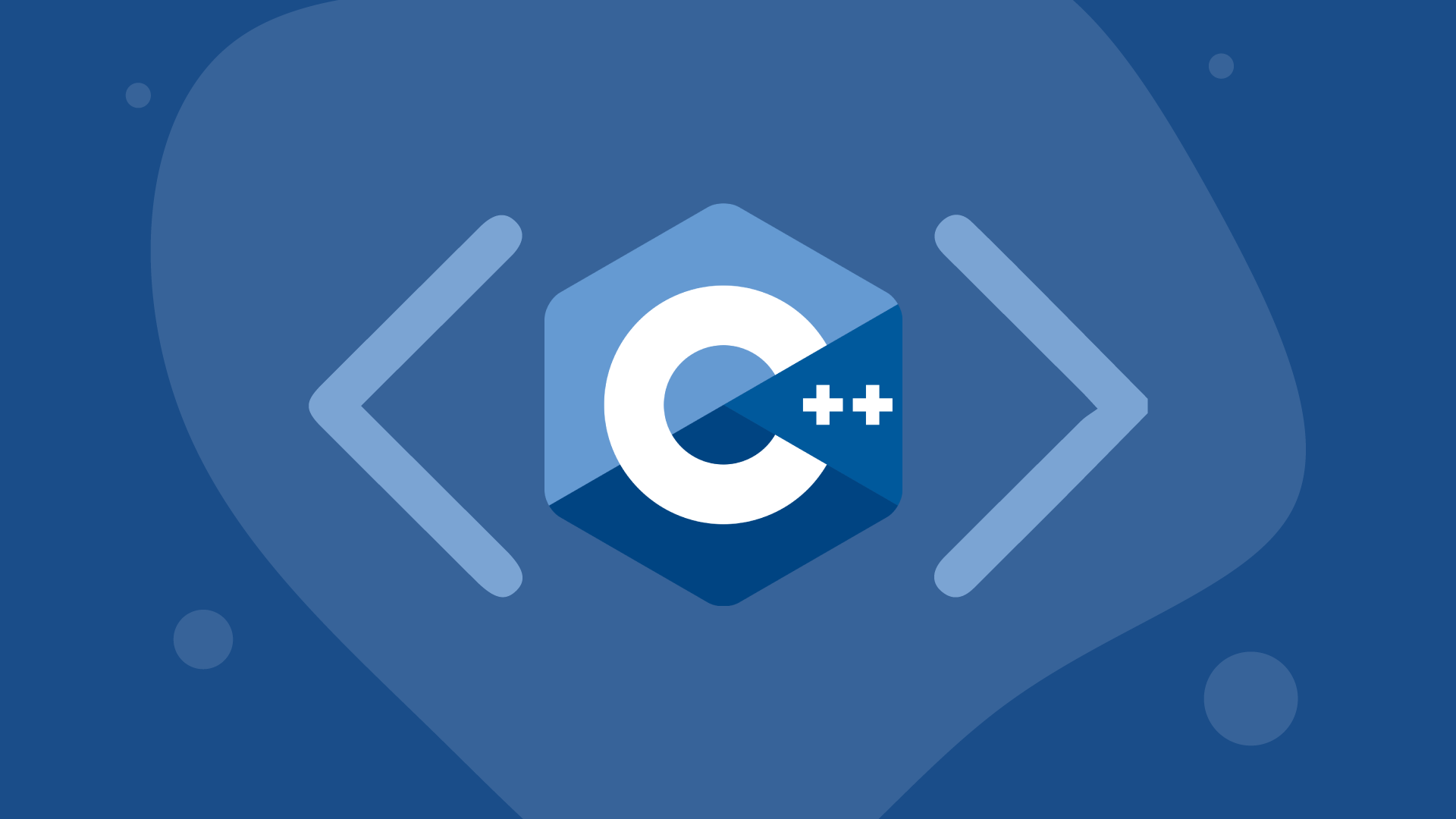
latch
- count기반의 스레드 동기화 도구
#include <iostream>
#include <mutex>
#include <latch>
#include <thread>
void foo(std::string name)
{
std::cout << "start work : " << name << std::endl;
std::cout << "finish work : " << name << std::endl;
// 세 스레드에서 집에가는 동작은 같이 하고 싶다면?
std::cout << "go home : " << name << std::endl;
}
int main()
{
std::jthread t1(foo, "kim"), t2(foo, "lee"), t3(foo, "park");
}
#include <iostream>
#include <mutex>
#include <latch>
#include <thread>
std::latch complete{3};
std::latch gohome{1};
void foo(std::string name)
{
std::cout << "start work : " << name << std::endl;
std::cout << "finish work : " << name << std::endl;
complete.count_down();
gohome.wait();
std::cout << "go home : " << name << std::endl;
}
int main()
{
std::jthread t1(foo, "kim"), t2(foo, "lee"), t3(foo, "park");
complete.wait(); // 카운트가 0일때까지 대기
gohome.count_down();
}
#include <iostream>
#include <mutex>
#include <latch>
#include <thread>
std::latch sync_point{3};
void foo(std::string name)
{
std::cout << "start work : " << name << std::endl;
std::cout << "finish work : " << name << std::endl;
sync_point.arrive_and_wait(); // 카운트를 1줄이고, 카운트가 0일때까지 대기한다
std::cout << "go home : " << name << std::endl;
sync_point.arrive_and_wait(); // Error - latch는 1회만 쓸 수 있다 여러번 쓰려면 barrier를 쓰자
}
int main()
{
std::jthread t1(foo, "kim"), t2(foo, "lee"), t3(foo, "park");
}
barrier
#include <iostream>
#include <mutex>
#include <barrier>
#include <thread>
void oncomplete()
{
std::cout << "oncomplete" << name << std::endl;
}
std::barrier sync_point{3, oncomplete}; // 콜백함수도 선언가능
void foo(std::string name)
{
std::cout << "start work : " << name << std::endl;
std::cout << "finish work : " << name << std::endl;
sync_point.arrive_and_wait();
std::cout << "go home : " << name << std::endl;
sync_point.arrive_and_wait(); // 2회 써도 무방
}
int main()
{
std::jthread t1(foo, "kim"), t2(foo, "lee"), t3(foo, "park");
}