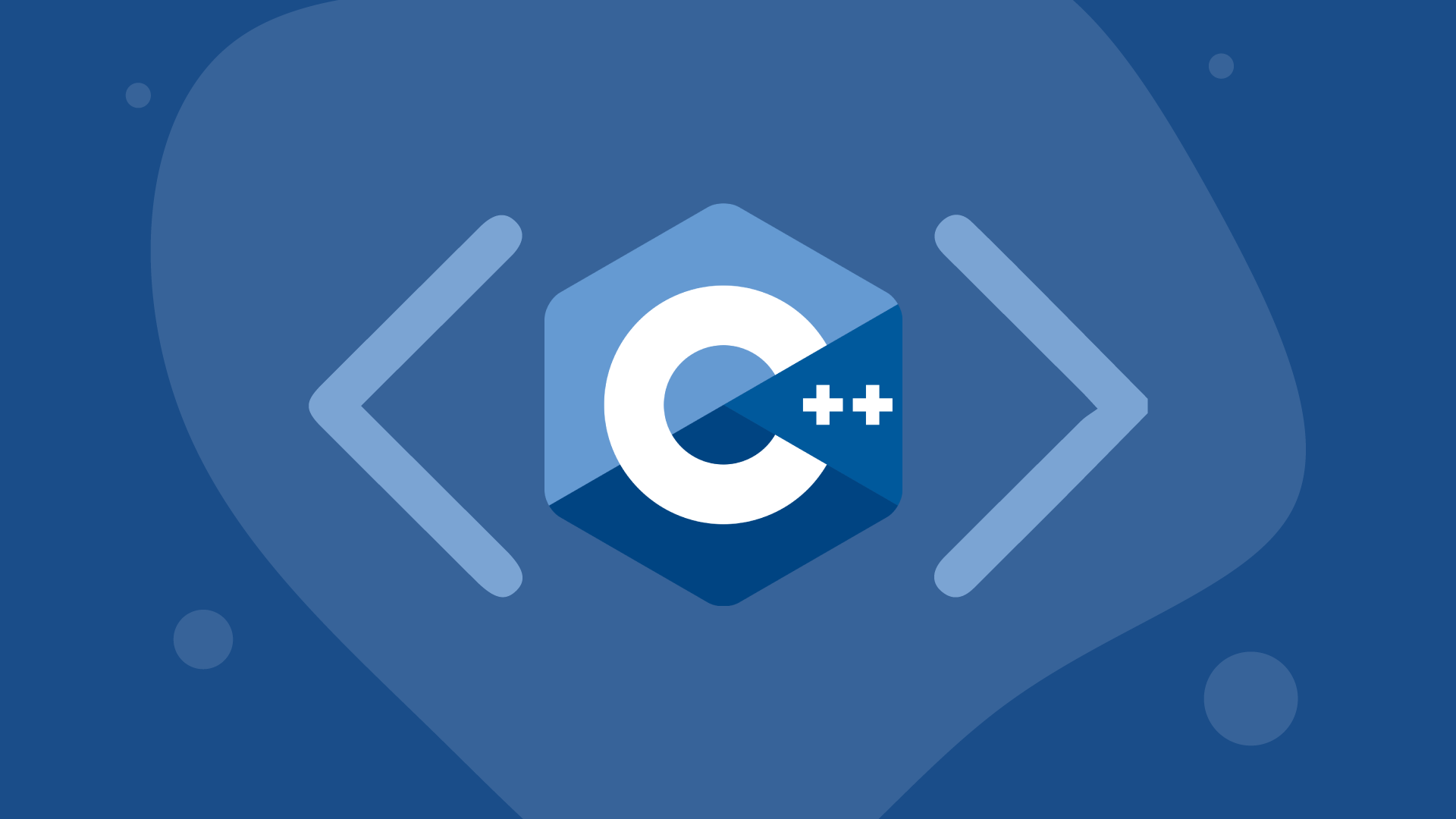
- 비동기 : 순차적으로 실행되진 않는다.
- 입출력(I/O) 작업(네트워크 작업)
- 내부적으로 복잡한 연산을 작업
-
멀티쓰레드 : 동시에 여러작업이 가능하다.
- C++에서 쓰레드 만들기
std::thread
std::jthread
(C++20)std::async
#include <iostream>
#include <thread>
#include <chrono>
#include <future>
using namespace std::literals;
int add(int a, int b)
{
std::this_thread::sleep_for(2s);
return a+b;
}
int main()
{
// add(10, 20); // 동기
std::future<int> ft = std::async(add, 10, 20); // 비동기
int ret = ft.get();
std::cout << "result : " << ret << std::endl;
}
#include <iostream>
#include <thread>
#include <chrono>
#include <future>
using namespace std::literals;
int add(int a, int b)
{
std::cout << "add : " << std::this_thread::get_id() << std::endl;
std::this_thread::sleep_for(2s);
return a+b;
}
int main()
{
// std::launch::async : 비동기로 만들어 주세요
std::future<int> ft = std::async(std::launch::async, add, 10, 20);
// std::launch::deferred : 지연된 실행으로 만들어 주세요(get에서 실행해 주세요)
std::future<int> ft = std::async(std::launch::deferred, add, 10, 20);
// std::launch::async | std::launch::deferred : 비동기 지연된 실행으로 해주세요
std::future<int> ft = std::async(std::launch::async | std::launch::deferred, add, 10, 20);
// 환경에 따라 달라진다(대부분은 지연된 실행에 비동기)
std::future<int> ft = std::async(add, 10, 20);
std::cout << "continue main : " << std::this_thread::get_id() << std::endl;
std::this_thread::sleep_for(2s);
int ret = ft.get();
std::cout << "result : " << ret << std::endl;
}
#include <iostream>
#include <thread>
#include <chrono>
#include <future>
using namespace std::literals;
int add(int a, int b)
{
std::cout << "start add" << std::endl;
std::this_thread::sleep_for(2s);
std::cout << "finish add" << std::endl;
return a+b;
}
int main()
{
std::future<int> ft = std::async(std::launch::async, add, 10, 20);
std::cout << "continue main" << std::endl;
// int ret = ft.get();
// get을 호출안한다면
// add는 호출이 되지 않을까??
// Nope! -> future의 소멸자에서 get이 자동호출되어 add가 호출되게 된다.
}