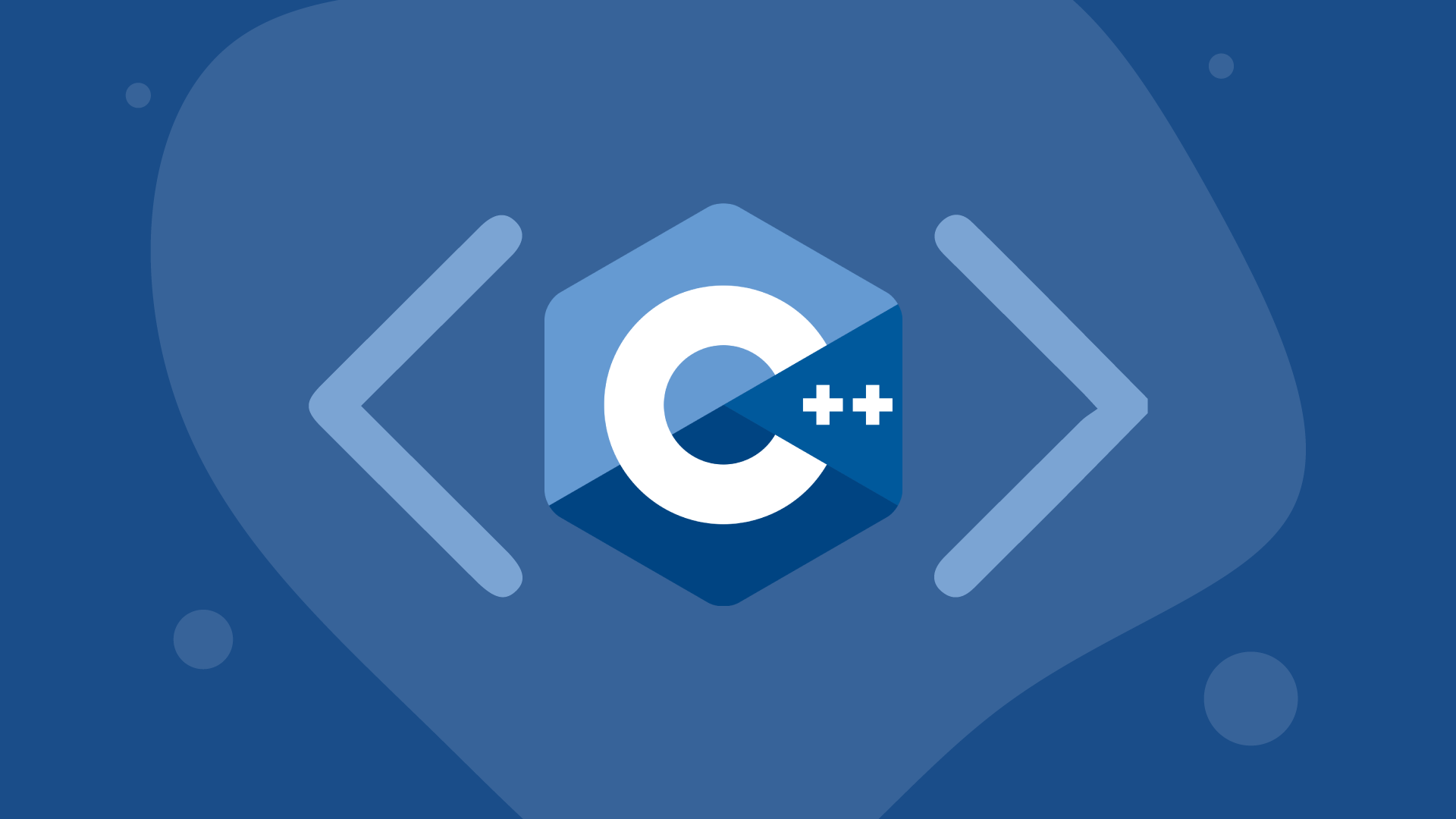
How to launch a thread (std::thread사용하기)
#include <iostream>
#include <thread>
void func1()
{
std::cout << "Hello from function \n";
}
class my_class {
public:
void operator()()
{
std::cout << "hello from the class with function call operator \n";
}
};
int main()
{
// 1. function으로 thread 생성
std::thread thread1(func1);
// 2. class operator로 thread 생성
my_class mc;
std::thread thread2(mc);
// 3. lambda로 thread 생성
std::thread thread3([] {
std::cout << "hello from the lambda \n";
});
thread1.join();
thread2.join();
thread3.join();
std::cout << "This is main thread \n";
}
hello from the class with function call operator
Hello from function
hello from the lambda
This is main thread
추가) sleep사용하기
#include <iostream>
#include <thread>
#include <chrono>
using namespace std;
int main()
{
thread::id id = this_thread::get_id();
cout << id << endl;
this_thread::sleep_for(3s);
this_thread::sleep_until(chrono::system_clock::now()+3s);
this_thread::yield();
}
joinabiligy of threads
#include <iostream>
#include <thread>
void func_1()
{
std::cout << "hello from method \n";
}
int main()
{
std::thread thread_1(func_1);
// 이 thread의 시작 시점이 궁금할 수 있다
// 생성과 동시에 바로 시작됨을 기억하자(join이 호출돼야 실행되는게 아님)
if (thread_1.joinable())
std::cout << "this is joinable thread \n";
// 여기서 join시 joinable == false
//thread_1.join();
if (thread_1.joinable())
{
std::cout << "this is joinable thread \n";
}
else
{
std::cout << "after calling join, thread_1 is not a joinable thread \n";
}
std::cout << "hello from main thread \n";
}
join and detach functions
detach
- 오브젝트와 스레드를 해제한다.
- detach 함수는 thread 오브젝트에 연결된 스레드를 떼어냅니다.
- 그래서 detach 이후에는 thread 오브젝트로 스레드를 제어할 수 없습니다
- 그러나 detach를 호출했다고 해서 관련된 스레드가 종료 되는 것이 아닙니다
- 즉 thread 오브젝트와 스레드의 연결이 끊어지는 것입니다.
#include <iostream>
#include <thread>
#include <chrono>
void func_1()
{
std::this_thread::sleep_for(std::chrono::milliseconds(5000));
std::cout << "hello from func_1 \n";
}
int main()
{
std::thread thread_1(func_1);
thread_1.join();
// thread_1.detach(); // detach시 func_1()은 불리지 않는다.
std::cout << "hello from main thread \n";
}
// ...
first.detach();
// 스레드 아이디가 초기화 된다.
std::cout << "after detach first thread id : " << first.get_id() << endl;
// 스레드가 멈출때 까지 대기
if (true == first.joinable()) // detach후 joinable하지 않으면 에러 발생
first.join();
if (true == second.joinable())
second.join();
// ...