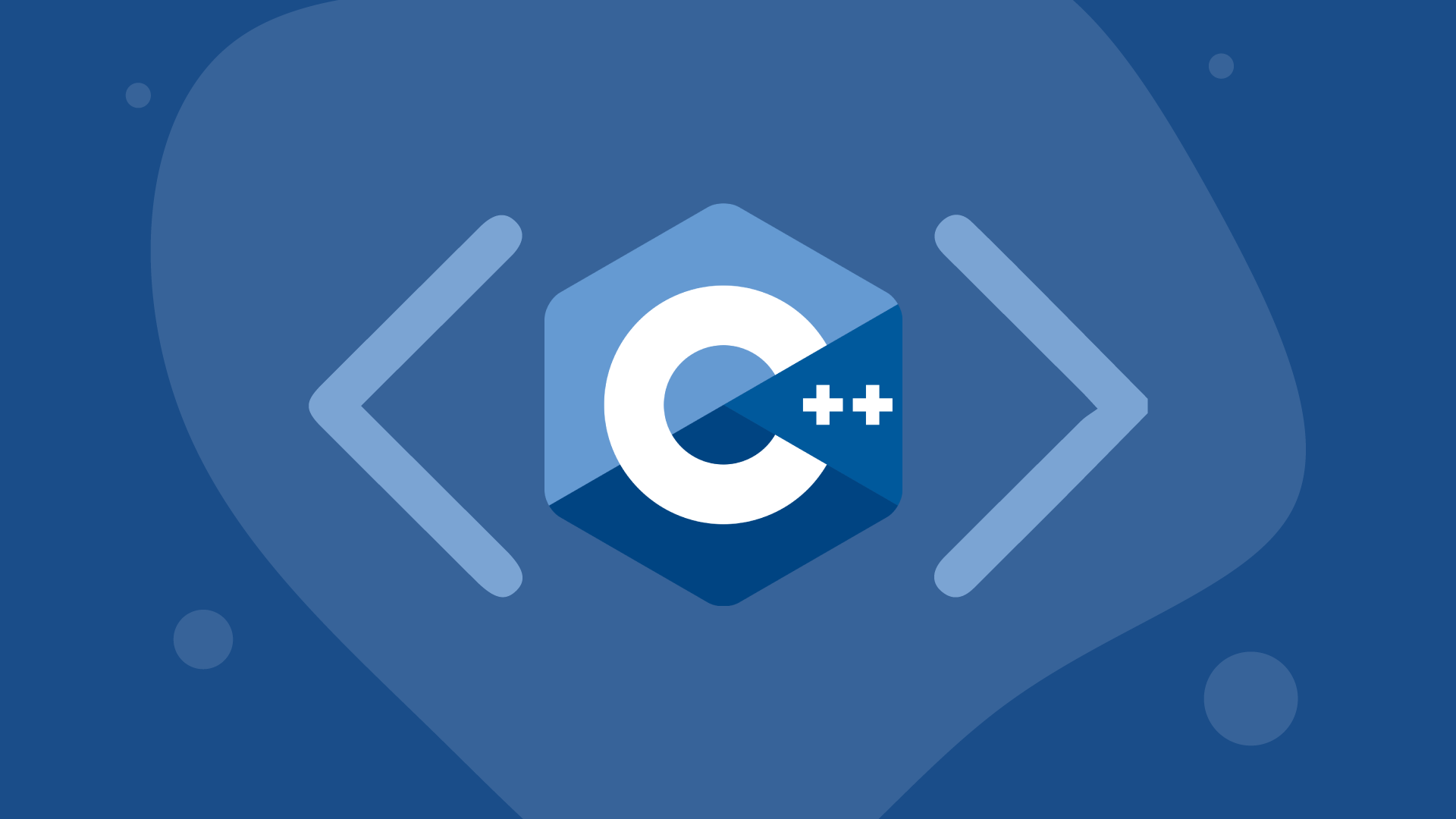
메모리 풀(pool)? - 메모리를 크게 미리 할당해서 사용
# 우선 우리는 메모리 풀을 이렇게 만들 것이다.
{ [32 32 ...][64 64 64 ...][128 128 ...][256 ...] }
# 메모리를 특정단위로 나누고
# 메모리할당이 필요한 오브젝트가 자신의 메모리크기에 맞는 메모리를 선택하게 할 것!
struct MemoryHeader
{
// [MemoryHeader][Data]
MemoryHeader(int32 size) : allocSize(size) { }
static void* AttachHeader(MemoryHeader* header, int32 size)
{
new(header)MemoryHeader(size); // placement new
return reinterpret_cast<void*>(++header); // ++은 메모리 헤더만큼 건너뛰어달란 말.
}
// 헤더주소를 보내주세요
static MemoryHeader* DetachHeader(void* ptr)
{
MemoryHeader* header = reinterpret_cast<MemoryHeader*>(ptr) - 1;
return header;
}
int32 allocSize; // 할당된 메모리 크기를 갖고있는다.
// TODO : 필요한 추가 정보
};
class MemoryPool
{
public:
MemoryPool(int32 allocSize);
~MemoryPool();
void Push(MemoryHeader* ptr); // 할당은 Push
MemoryHeader* Pop(); // 사용은 Pop
private:
int32 _allocSize = 0; // 얼마만큼 메모리를 할당할 것인가
atomic<int32> _allocCount = 0; // 몇개가 할당되었나
USE_LOCK;
queue<MemoryHeader*> _queue;
};
MemoryPool::MemoryPool(int32 allocSize) : _allocSize(allocSize)
{
}
MemoryPool::~MemoryPool()
{
while (_queue.empty() == false)
{
MemoryHeader* header = _queue.front();
_queue.pop();
::free(header);
}
}
void MemoryPool::Push(MemoryHeader* ptr)
{
WRITE_LOCK;
ptr->allocSize = 0;
// Pool에 메모리 반납
_queue.push(ptr);
_allocCount.fetch_sub(1);
}
MemoryHeader* MemoryPool::Pop()
{
MemoryHeader* header = nullptr;
{
WRITE_LOCK;
// Pool에 여분이 있는지?
if (_queue.empty() == false)
{
// 있으면 하나 꺼내온다
header = _queue.front();
_queue.pop();
}
}
// 없으면 새로 만들다
if (header == nullptr)
{
header = reinterpret_cast<MemoryHeader*>(::malloc(_allocSize));
}
else
{
ASSERT_CRASH(header->allocSize == 0);
}
_allocCount.fetch_add(1);
return header;
}
메모리를 어떻게 관리할 것인가
class Memory
{
enum
{
// ~1024까지 32단위, ~2048까지 128단위, ~4096까지 256단위
POOL_COUNT = (1024 / 32) + (1024 / 128) + (2048 / 256),
MAX_ALLOC_SIZE = 4096
};
public:
Memory();
~Memory();
void* Allocate(int32 size);
void Release(void* ptr);
private:
vector<MemoryPool*> _pools;
// 메모리 크기 <-> 메모리 풀
// O(1) 빠르게 찾기 위한 테이블
MemoryPool* _poolTable[MAX_ALLOC_SIZE + 1];
};
Memory::Memory()
{
int32 size = 0;
int32 tableIndex = 0;
for (size = 32; size <= 1024; size += 32)
{
MemoryPool* pool = new MemoryPool(size);
_pools.push_back(pool);
while (tableIndex <= size)
{
_poolTable[tableIndex] = pool;
tableIndex++;
}
}
for (; size <= 2048; size += 128)
{
MemoryPool* pool = new MemoryPool(size);
_pools.push_back(pool);
while (tableIndex <= size)
{
_poolTable[tableIndex] = pool;
tableIndex++;
}
}
for (; size <= 4096; size += 256)
{
MemoryPool* pool = new MemoryPool(size);
_pools.push_back(pool);
while (tableIndex <= size)
{
_poolTable[tableIndex] = pool;
tableIndex++;
}
}
}
Memory::~Memory()
{
for (MemoryPool* pool : _pools)
delete pool;
_pools.clear();
}
void* Memory::Allocate(int32 size)
{
MemoryHeader* header = nullptr;
const int32 allocSize = size + sizeof(MemoryHeader);
if (allocSize > MAX_ALLOC_SIZE)
{
// 메모리 풀링 최대 크기를 벗어나면 일반 할당
header = reinterpret_cast<MemoryHeader*>(::malloc(allocSize));
}
else
{
// 메모리 풀에서 꺼내온다
header = _poolTable[allocSize]->Pop();
}
return MemoryHeader::AttachHeader(header, allocSize);
}
void Memory::Release(void* ptr)
{
MemoryHeader* header = MemoryHeader::DetachHeader(ptr);
const int32 allocSize = header->allocSize;
ASSERT_CRASH(allocSize > 0);
if (allocSize > MAX_ALLOC_SIZE)
{
// 메모리 풀링 최대 크기를 벗어나면 일반 해제
::free(header);
}
else
{
// 메모리 풀에 반납한다
_poolTable[allocSize]->Push(header);
}
}
그런데 Stomp쓰면 되지 이걸 왜??
Stomp는 메모리 반환시 메모리를 커널단에서 날려버린다.
메모리 할당 해제가 많을경우 오히려 손해일 수 있다.