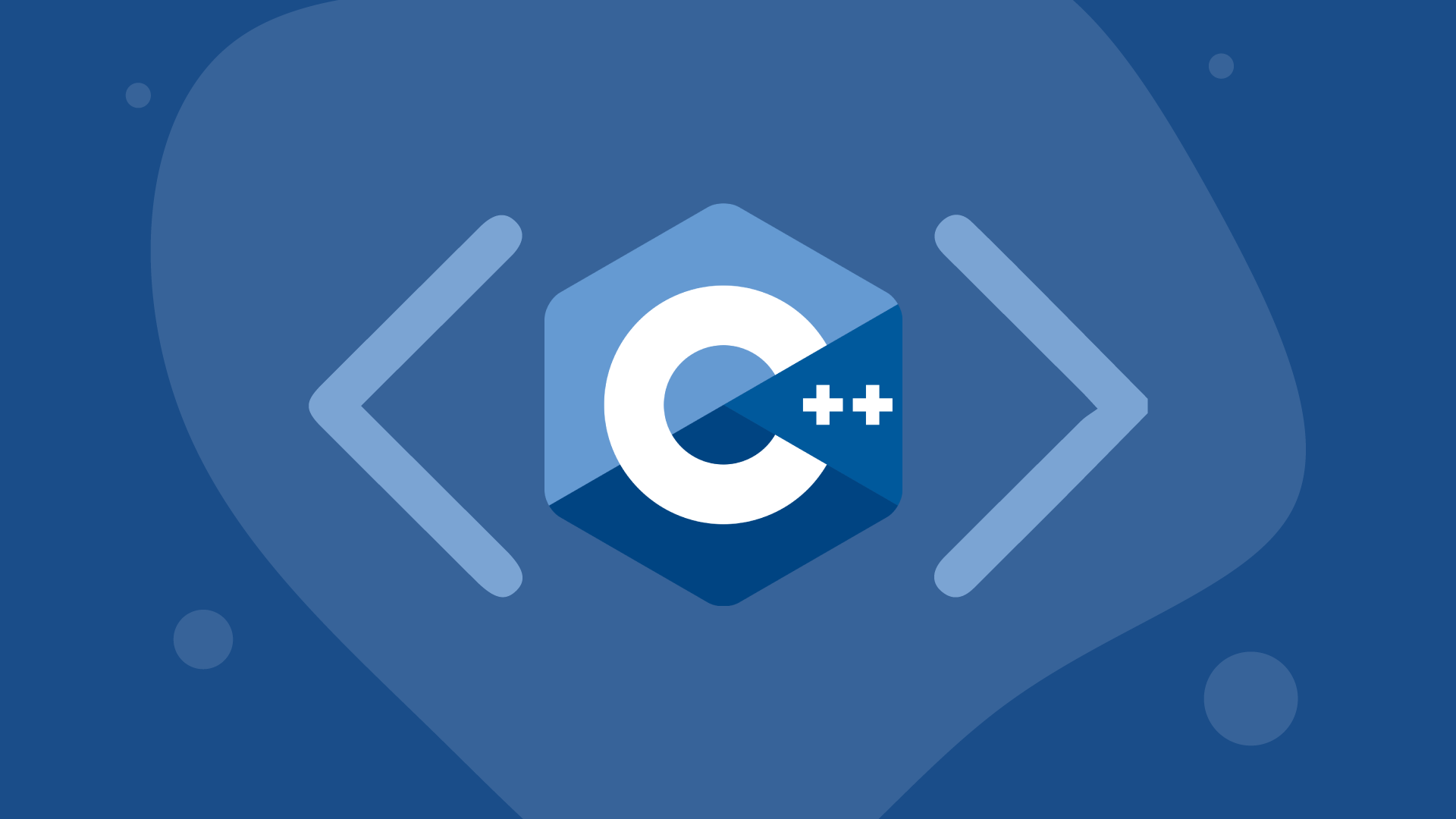
// STL의 vector를 생각해 보자.
template<typename T> class vector
{
T* buff;
public:
void resize(int n)
{
// 버퍼 재할당이 필요하다면?
// new?, malloc?, calloc? ... 뭘쓰나??
// STL내부에는 메모리할당기를 제공한다.
}
};
int main()
{
vector<int> v(10);
v.resize(20);
}
template<typename T> class allocator
{
public:
T* allocator() {}
void deallocate() {}
};
template<typename T, typename Ax = allocator<T>> class vector
{
T* buff;
Ax ax;
public:
void resize(int n)
{
T* p = ax.allocate(n);
ax.deallocate(p);
}
};
int main()
{
vector<int, MyAlloc<int>> v(10); // 이런 선언이 가능하다.
v.resize(20);
}
rebind
template<typename T> class allocator
{
public:
T* allocate(int sz) { return new T[sz]; }
void deallocate(T* p) { delete[] p; }
tempate<typename U> struct rebind
{
typename allocator<U> other;
}
};
// rebind
template<typename T, typename Ax = allocator<T>> class list
{
struct NODE
{
T data;
NODE* next, *prev;
};
//Ax ax; // allocator<int> 버전임
// 필요한거는 allocator<NODE>가 필요하다.
// allocator<int>::rebind<NODE>::other ax;
// allocator<NODE> ax;
typename Ax::template rebind<NODE>::other ax;
public:
void push_front(const T& a)
{
ax.allocate(1);
}
};
int main()
{
list<int> s;
s.push_front(10);
}