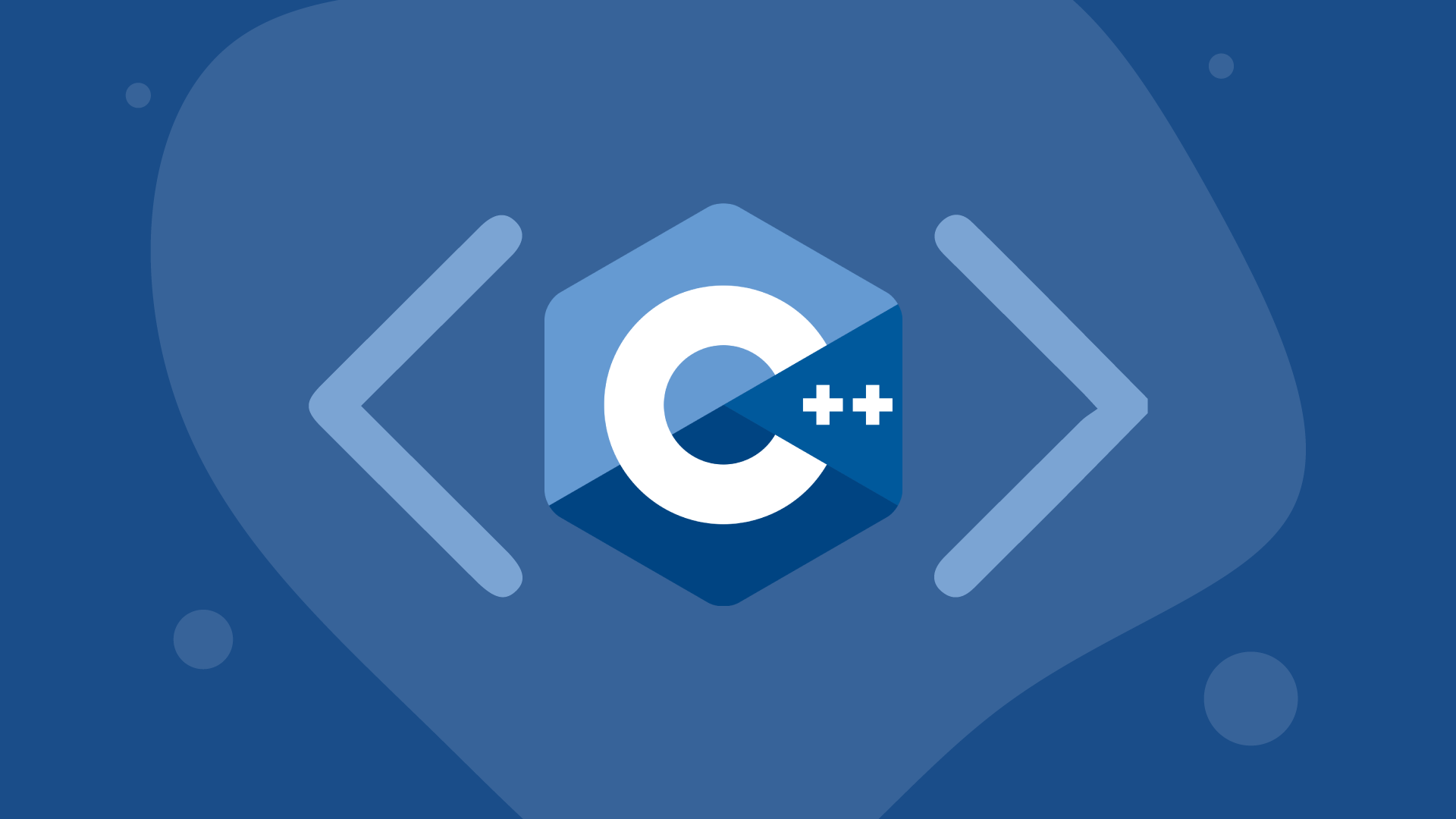
Bridge Pattern
구현과 추상화 개념을 분리해서 각각을 독립적으로 변형할 수 있게한다.
역시 코드로 …
#include <iostream>
using namespace std;
struct IMP3
{
virtual void Play() = 0;
virtual void Stop() = 0;
virtual ~IMP3() {}
}
class IPod
{
public:
void Play() { cout << "Play MP3" << endl; }
void Stop() { cout << "Stop MP3" << endl; }
};
class People
{
public:
void UseMP3(IMP3* p)
{
p->Play();
p->PlayOneMinute(); // 이 함수를 추가하고 싶다면?
}
};
int main()
{
}
class MP3
{
IMP3* pImpl;
public:
MP3()
{
pImpl = new IPod;
}
void Play() { pImpl->Play(); }
void Stop() { pImpl->Stop(); }
void PlayOneMinute() {
// 1분 후 종료
}
};
class People
{
public:
void UseMP3(MP3* p)
{
p->Play();
p->PlayOneMinute(); // MP3에 이 함수를 만들면된다.
}
};
PIMPL IDioms
// Point.h
class Point
{
int x, y;
public:
Point (int a = 0, int b = 0);
void Print() const;
};
// Point.cpp
#include "Point.h"
Point::Point(int a, int b) : x(a), y(b) {}
void Point::Print() const
{
cout << x << ", " << y << endl;
}
// main
#include "Point.h"
int main()
{
Point p(1, 2);
p.Print();
}
문제) #include "Point.h"
는 수백 수천개에서 인클루드 한다면?
재컴파일하는데 엄청난 시간이 요구될 것이다. -> 개선해보자.
// PointImpl.h
class PointImpl
{
int x, y;
public:
PointImpl(int a = 0, int b = 0);
void Print() const;
};
// PointImpl.cpp
PointImpl::PointImpl(int a, int b) : x(a), y(b) {}
void PointImpl::Print() const
{
cout << x << ", " << y << endl;
}
// Point.h
class PointImpl;
class Point
{
PointImpl* pImpl;
public:
Point(int a= 0, int b = 0);
void Print() const;
};
// Point.cpp
Point::Point(int a, int b)
{
pImpl = new PointImpl(a, b);
}
void Point::Print() const
{
pImpl->Print();
}
PointImpl
에서 실제 동작을하고 수정을 해도 인클루드는 Point에서 하기에 디버깅에 영향을 주지 않는다. 이를 PIMPL(Pointer to IMPlementation)이라한다.
- 컴파일 속도를 향싱
- 완벽한 정보 은닉이 가능, 헤더파일을 감출수 있다.