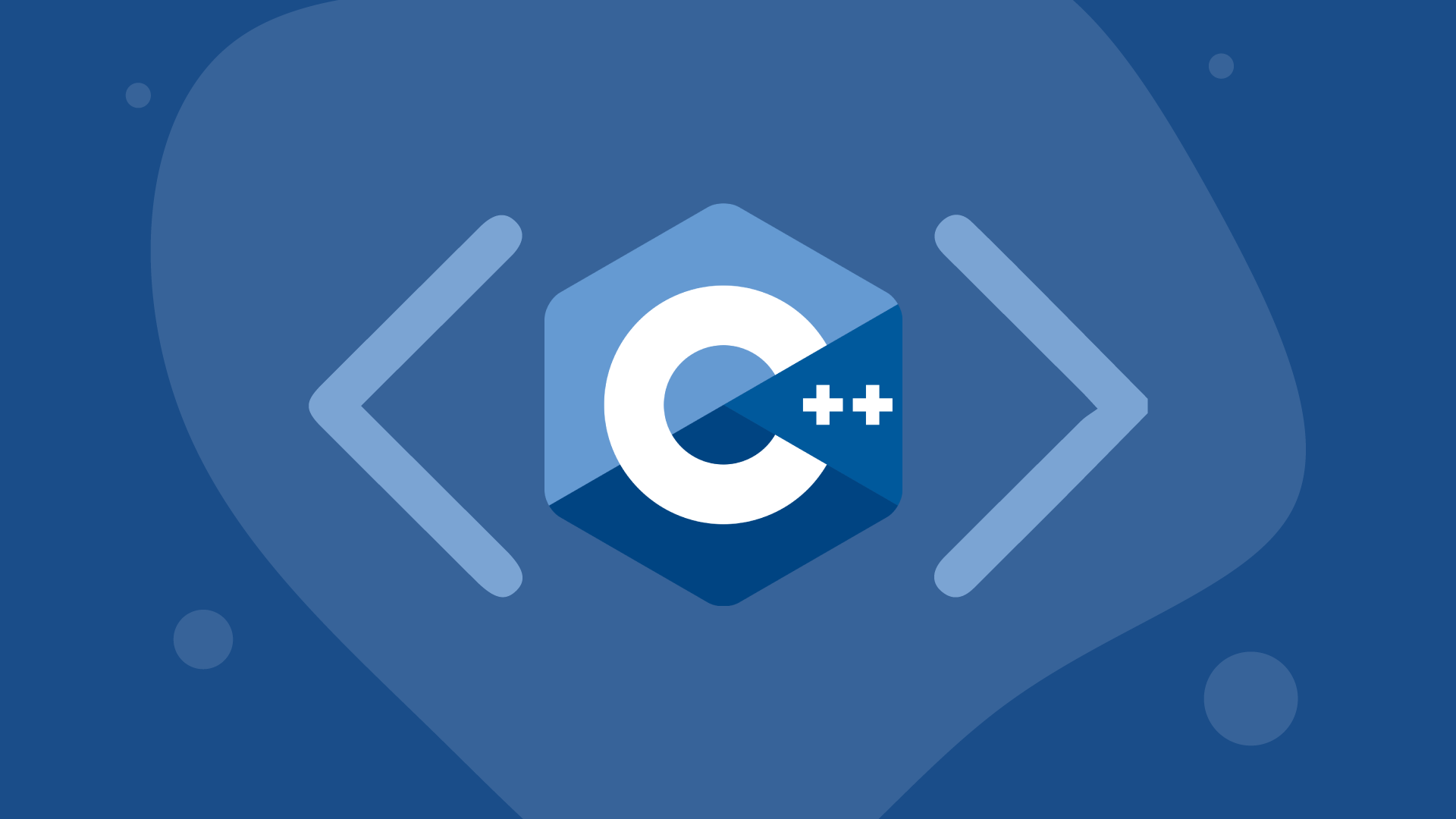
#include <iostream>
#include <algorithm>
using namespace std;
void Sort(int* x, int sz)
{
for(int i = 0; i < sz-1; i++)
{
for(int j = i+1; j < sz;j++)
{
if(x[i] > x[j]) // 오름차순, 내림차순을 매번 코드를 변경함으로 수정해야하나??
swap(x[i], x[j]);
}
}
}
int main()
{
int x[10] = {1,3,5,7,9,2,4,6,8,10};
Sort(x,10);
for(auto n : x);
cout << n << ", ";
}
void Sort(int* x, int sz, bool(*cmp)(int, int))
{
for(int i = 0; i < sz-1; i++)
{
for(int j = i+1; j < sz;j++)
{
if( cmp(x[i], x[j]) )
swap(x[i], x[j]);
}
}
}
bool cmp1(int a, int b)
{
return a < b;
}
int main()
{
int x[10] = {1,3,5,7,9,2,4,6,8,10};
Sort(x,10,&cmp1);
for(auto n : x);
cout << n << ", ";
}
이것도 일종의 Strategy Pattern이 된다.
// 람다 표현식으로 수정가능.
Sort(x, 10, [](int a, int b){ return a>b; })
cmp의 호출이 잦다면 오버헤드의 원인이 되기도 한다. -> inline으로 변경해야한다.
template<typename T>
void Sort(int* x, int sz, T cmp)
{
for(int i = 0; i < sz-1; i++)
{
for(int j = i+1; j < sz;j++)
{
if( cmp(x[i], x[j]) )
swap(x[i], x[j]);
}
}
}
int main()
{
int x[10] = {1,3,5,7,9,2,4,6,8,10};
Sort(x, 10, [](int a, int b){ return a>b; })
// 람다표현식이 인라인화되면서 성능향상을 가져온다.
for(auto n : x);
cout << n << ", ";
}