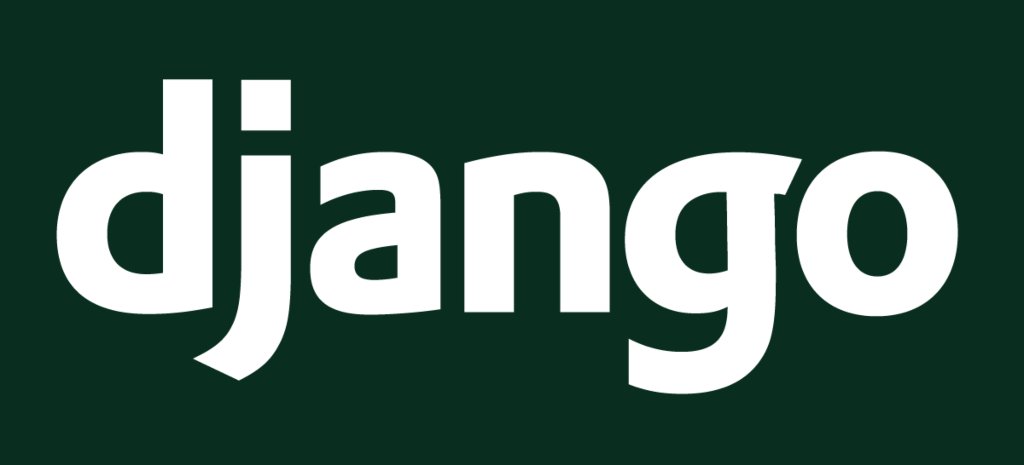
$ python manage.py startapp commentapp
# pragmatic\settings.py
# ...
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'bootstrap4',
'profileapp',
'accountapp',
'articleapp',
'commentapp',
]
# ...
# pragmatic\urlspy
# ...
urlpatterns = [
path('admin/', admin.site.urls),
path('accounts/', include('accountapp.urls')),
path('profiles/', include('profileapp.urls')),
path('articles/', include('articleapp.urls')),
path('comments/', include('commentapp.urls')),
] + static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
# commentapp\models.py
class Comment(models.Model):
article = models.ForeignKey(Article, on_delete=models.SET_NULL, null=True, related_name='comment')
writer = models.ForeignKey(User, on_delete=models.SET_NULL, null=True, related_name='comment')
content = models.TextField(null=False)
created_at = models.DateTimeField(auto_now=True)
# commentapp\formspy
class CommentCreationForm(ModelForm):
class Meta:
model = Comment
fields = ['content']
# commentapp\urls.py
app_name = 'commentapp'
urlpatterns = [
path('create/', CommentCreateView.as_view(), name='create'),
]
$ python manage.py makemigrations
$ python manage.py migrate
# commentapp\views.py
class CommentCreateView(CreateView):
model = Comment
form_class = CommentCreationForm
template_name = 'commentapp/create.html'
def get_success_url(self):
return reverse('articleapp:detail', kwargs={'pk': self.object.article.pk})
<!-- commentapp\create.html -->
{% extends 'base.html' %}
{% load bootstrap4 %}
{% block content %}
<div style="text-align: center; max-width: 500px; margin: 4rem auto">
<div class="mb-4">
<h4>Comment Create</h4>
</div>
<form action="{% url 'commentapp:create' %}" method="post" enctype="multipart/form-data">
{% csrf_token %}
{% bootstrap_form form %}
<input type="submit" class="btn btn-dark rounded-pill col-6 mt-3">
<input type="hidden" name="article_pk" value="">
</form>
</div>
{% endblock %}
commentapp을 게시글 아래 넣어보자
<!-- articleapp\detail.html -->
{% extends 'base.html' %}
{% block content %}
<div style="text-align: center; max-width: 700px; margin: 4rem auto">
<h1>
{{ target_article.title }}
</h1>
<h4>
{{ target_article.writer.profile.nickname }}
</h4>
<img style="width:100%; border-radius: 2rem;"
src="{{ target_article.image.url }}" alt="">
<p>
{{ target_article.content }}
</p>
{% if target_article.writer == user %}
<a href="{% url 'articleapp:update' pk=target_article.pk %}" class="btn btn-danger rounded-pill col-3">
Update
</a>
<a href="{% url 'articleapp:delete' pk=target_article.pk %}" class="btn btn-danger rounded-pill col-3">
Delete
</a>
<hr>
{% endif %}
{% include 'commentapp/create.html' with article=target_article %}
</div>
{% endblock %}
# articleapp\views.py
# ...
class ArticleDetailView(DetailView, FormMixin):
model = Article
form_class = CommentCreationForm
context_object_name = 'target_article'
template_name = 'articleapp/detail.html'
<!-- commentapp\create.html -->
{% load bootstrap4 %}
{% block content %}
<div style="text-align: center; max-width: 500px; margin: 4rem auto">
<div class="mb-4">
<h4>Comment Create</h4>
</div>
<form action="{% url 'commentapp:create' %}" method="post" enctype="multipart/form-data">
{% csrf_token %}
{% bootstrap_form form %}
{% if user.is_authenticated %}
<input type="submit" class="btn btn-dark rounded-pill col-6 mt-3">
{% else %}
<a href="{% url 'accountapp:login' %}?next={{request.path}}" class="btn btn-dark rounded-pill col-6 mt-3">
Login
</a>
{% endif %}
<input type="hidden" name="article_pk" value="{{article.pk}}">
</form>
</div>
{% endblock %}
# commentapp\view.py
def form_valid(self, form):
temp_comment = form.save(commit=False)
temp_comment.article = Article.object.get(pk=self.request.POST['article_pk'])
temp_comment.writer = self.request.user
temp_comment.save()
return super().form_valid(form)
def get_success_url(self):
return reverse('articleapp:detail', kwargs={'pk': self.object.article.pk})