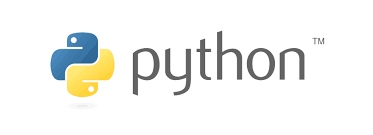
일자별 거래가 확인
import pybithumb
btc = pybithumb.get_ohlcv("BTC")
print(btc)
open high ... close volume
time ...
2013-12-27 00:00:00 737000.0 755000.0 ... 755000.0 3.780000
2013-12-28 00:00:00 750000.0 750000.0 ... 750000.0 12.000000
2013-12-29 00:00:00 750000.0 750000.0 ... 739000.0 19.058000
2013-12-30 00:00:00 740000.0 772000.0 ... 768000.0 9.488973
2013-12-31 00:00:00 768000.0 800000.0 ... 768000.0 18.650350
... ... ... ... ... ...
2021-11-10 00:00:00 80641000.0 82445000.0 ... 81806000.0 4036.842562
2021-11-11 00:00:00 81807000.0 82198000.0 ... 79080000.0 4876.734224
2021-11-12 00:00:00 79100000.0 79280000.0 ... 77546000.0 4152.614555
2021-11-13 00:00:00 77528000.0 78736000.0 ... 78668000.0 2536.074419
2021-11-14 20:00:00 78684000.0 79044000.0 ... 78270000.0 1825.122086
일자별 종가 확인
import pybithumb
btc = pybithumb.get_ohlcv("BTC")
close = btc[‘close’])
print(close)
time
2013-12-27 00:00:00 755000.0
2013-12-28 00:00:00 750000.0
2013-12-29 00:00:00 739000.0
2013-12-30 00:00:00 768000.0
2013-12-31 00:00:00 768000.0
...
2021-11-10 00:00:00 81806000.0
2021-11-11 00:00:00 79080000.0
2021-11-12 00:00:00 77546000.0
2021-11-13 00:00:00 78668000.0
2021-11-14 20:00:00 78264000.0
Name: close, Length: 2790, dtype: float64
5일 이동병균선 확인
import pybithumb
btc = pybithumb.get_ohlcv("BTC")
close = btc[‘close’])
print((close[0] + close[1] + close[2] + close[3] + close[4]) / 5)
print((close[1] + close[2] + close[3] + close[4] + close[5]) / 5)
print((close[2] + close[3] + close[4] + close[5] + close[6]) / 5)
# 이렇게 구현해도 되지만 pybithumb에 이미 구현이 되어 있음
import pybithumb
btc = pybithumb.get_ohlcv("BTC")
close = btc[‘close’]
window = close.rolling(5)
ma5 = window.mean()
print(ma5)
종가 5일 이동평균선을 이용해 상승/하락장 구분하기
import pybithumb
df = pybithumb.get_ohlcv("BTC")
# 종가 5일의 평균
ma5 = df['close'].rolling(window=5).mean()
# 전일의 (종가 5일)이동 평균선
last_ma5 = ma5[-2] # 참고로 -2는 -> ... [마지막-1 <-- 여기임][마지막]
price = pybithumb.get_current_price("BTC")
if price > last_ma5:
print("상승장")
else:
print("하락장")
그냥 함수로 만들어 보자
import pybithumb
def bull_market(ticker):
df = pybithumb.get_ohlcv(ticker)
ma5 = df['close'].rolling(window=5).mean()
price = pybithumb.get_current_price(ticker)
last_ma5 = ma5[-2]
if price > last_ma5:
return True
else:
return False
'''
is_bull = bull_market("BTC")
if is_bull :
print("상승장")
else:
print("하락장")
'''
# 모든 티커에 대해 확인해 보자
tickers = pybithumb.get_tickers()
for ticker in tickers:
is_bull = bull_market(ticker)
if is_bull:
print(ticker, " 상승장")
else:
print(ticker, " 하락장")
Qt를 이용해 상승/하락장 알리미 만들기
Table Widget을 추가하고 행을 위 그림과 같이 추가
import sys
from PyQt5.QtWidgets import *
from PyQt5 import uic
form_class = uic.loadUiType("./bullMargetTester.ui")[0]
class MyWindow(QMainWindow, form_class):
def __init__(self):
super().__init__()
self.setupUi(self)
app = QApplication(sys.argv)
win = MyWindow()
win.show()
app.exec_()
여기까지 완료 후 실행
5초 마다 티커 받기
import sys
from PyQt5.QtWidgets import *
from PyQt5 import uic
from PyQt5.QtCore import *
tickers = ["BTC", "ETH", "BCH", "ETC"]
form_class = uic.loadUiType("./bullMargetTester.ui")[0]
class MyWindow(QMainWindow, form_class):
def __init__(self):
super().__init__()
self.setupUi(self)
timer = QTimer(self)
timer.start(5000)
timer.timeout.connect(self.timeout)
def timeout(self):
for i, ticker in enumerate(tickers):
item = QTableWidgetItem(ticker)
self.tableWidget.setItem(i, 0, item)
app = QApplication(sys.argv)
window = MyWindow()
window.show()
app.exec_()
나머지 데이터 추출
import sys
import pybithumb
from PyQt5.QtWidgets import *
from PyQt5 import uic
from PyQt5.QtCore import *
tickers = ["BTC", "ETH", "BCH", "ETC"]
form_class = uic.loadUiType("./bullMargetTester.ui")[0]
class MyWindow(QMainWindow, form_class):
def __init__(self):
super().__init__()
self.setupUi(self)
timer = QTimer(self)
timer.start(5000)
timer.timeout.connect(self.timeout)
def get_market_infos(self, ticker):
df = pybithumb.get_ohlcv(ticker)
ma5 = df['close'].rolling(window=5).mean()
last_ma5 = ma5[-2]
price = pybithumb.get_current_price(ticker)
state = None
if price > last_ma5:
state = "상승장"
else:
state = "하락장"
return price, last_ma5, state
def timeout(self):
for i, ticker in enumerate(tickers):
item = QTableWidgetItem(ticker)
self.tableWidget.setItem(i, 0, item)
price, last_ma5, state = self.get_market_infos(ticker)
self.tableWidget.setItem(i, 1, QTableWidgetItem(str(price)))
self.tableWidget.setItem(i, 2, QTableWidgetItem(str(last_ma5)))
self.tableWidget.setItem(i, 3, QTableWidgetItem(state))
app = QApplication(sys.argv)
window = MyWindow()
window.show()
app.exec_()