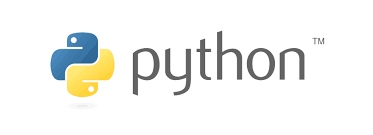
코루틴?
import asyncio
async def make_americano():
print("Americano Start")
await asyncio.sleep(3)
print("Americano End")
async def make_latte():
print("Latte Start")
await asyncio.sleep(5)
print("Latte End")
async def main():
coro1 = make_americano()
coro2 = make_latte()
await asyncio.gather(
coro1,
coro2
)
print("Main Start")
asyncio.run(main())
print("Main End")
Main Start
Americano Start
Latte Start
Americano End
Latte End
Main End
BitHumb 웹소켓
간단하게 응답 받아보기
import websockets
import asyncio
async def bithumb_ws_client():
uri = "wss://pubwss.bithumb.com/pub/ws"
# ??? - 이런 문법은 처음인데?
async with websockets.connect(uri) as websocket:
greeting = await websocket.recv()
print(greeting)
async def main():
await bithumb_ws_client()
asyncio.run(main())
async with ~ as ~
f = open('path/to/open', 'w')
f.write("hello world")
f.close()
# 위 문법을 간단하게 아래 처럼 표기가능
with open('path/to/open', 'w') as f
f.write("hello world")
# f의 close는 알아서 호출해준다.
콘솔창에 웹소켓을 이용하여 코인정보 출력
import websockets
import asyncio
import json
async def bithumb_ws_client():
uri = "wss://pubwss.bithumb.com/pub/ws"
async with websockets.connect(uri, ping_interval=None) as websocket:
greeting = await websocket.recv()
print(greeting)
subscribe_fmt = {
"type":"ticker",
"symbols": ["BTC_KRW"],
"tickTypes": ["1H"]
}
subscribe_data = json.dumps(subscribe_fmt)
await websocket.send(subscribe_data)
while True:
data = await websocket.recv()
data = json.loads(data)
print(data)
async def main():
await bithumb_ws_client()
asyncio.run(main())
아래처럼 들어온다
{"status":"0000","resmsg":"Connected Successfully"}
{'status': '0000', 'resmsg': 'Filter Registered Successfully'}
{'type': 'ticker', 'content': {'tickType': '1H', 'date': '20211116', 'time': '083614', 'openPrice': '77409000', 'closePrice': '77670000', 'lowPrice': '77409000', 'highPrice': '77850000', 'value': '5933047009.11453', 'volume': '76.38922442', 'sellVolume': '20.47325965', 'buyVolume': '55.91596477', 'prevClosePrice': '78363000', 'chgRate': '0.34', 'chgAmt': '261000', 'volumePower': '273.12', 'symbol': 'BTC_KRW'}}
{'type': 'ticker', 'content': {'tickType': '1H', 'date': '20211116', 'time': '083616', 'openPrice': '77409000', 'closePrice': '77679000', 'lowPrice': '77409000', 'highPrice': '77850000', 'value': '5934041300.31453', 'volume': '76.40202442', 'sellVolume': '20.48605965', 'buyVolume': '55.91596477', 'prevClosePrice': '78363000', 'chgRate': '0.35', 'chgAmt': '270000', 'volumePower': '272.95', 'symbol': 'BTC_KRW'}}
...
from pybithumb import WebSocketManager
import sys
from PyQt5.QtWidgets import *
from PyQt5.QtCore import *
from PyQt5.QtGui import QIcon
import time
class Worker(QThread):
recv = pyqtSignal(str)
def run(self):
# create websocket for Bithumb
wm = WebSocketManager("ticker", ["BTC_KRW"])
while True:
data = wm.get()
self.recv.emit(data['content']['closePrice'])
class MyWindow(QMainWindow):
def __init__(self):
super().__init__()
label = QLabel("BTC", self)
label.move(20, 20)
self.price = QLabel("-", self)
self.price.move(80, 20)
self.price.resize(100, 20)
button = QPushButton("Start", self)
button.move(20, 50)
button.clicked.connect(self.click_btn)
self.th = Worker()
self.th.recv.connect(self.receive_msg)
@pyqtSlot(str)
def receive_msg(self, msg):
print(msg)
self.price.setText(msg)
def click_btn(self):
self.th.start()
if __name__ == '__main__':
app = QApplication(sys.argv)
mywindow = MyWindow()
mywindow.show()
app.exec_()